Triggers
Dynamically react and run flows based on advanced match criteria
Triggers are used to trigger and run a flow based on some external event meeting certain criteria.
A flow file typically consists of two main sections:
- the triggers associated with the flow, and
- the flow steps.
Below is an example of the triggers section:
triggers:
- keyword: order_return
action:
jump: start
data:
order_id: o-4
- regex: order.*debug
action:
jump: order_debug
From this snippet you can see that the triggers section can contain multiple trigger elements, and all trigger elements get evaluated every time a new event occurs.
So when the user types the text order debug
in their chat client, a Meya integration will create a new say
event. Once the event is created all the triggers will be evaluated at the same time, and the one with the best match will "fire" - in this case it will be the regex
trigger.
Action
By default, when a trigger matches it will start the flow at the first step. This behaviour can be customized by using the action
property. The action
property takes a component element, and will execute the specified component instead of the first step of the flow.
This allows you to use flow branching components such as jump
to jump to a specific label step in the flow.
Note, the trigger's action
property works the same as a button & quick reply's action
property.
When
The when
field allows you to customize when a trigger should be evaluated. Whenever the
app receives a new event all the configured triggers are loaded and only the triggers with when
conditions that are true will be evaluated.
This allows you to dynamically activate or deactivate triggers.
When is a trigger active?
By default triggers are evaluated when the following is true (this also applies to dynamic triggers):
- The event is not marked as sensitive, and
- The event is a user event (bot events should not cause triggers to evaluate), and
- The thread is in the bot mode (for example, all triggers are deactivated once the thread is in agent mode).
Warning
Setting
when: true
, will cause the trigger to always be evaluated, even for bot events. Only do this if you're very confident that your matching criteria makes sense and won't always run the flow.
Reference documentation
The Meya SDK provides many different types of triggers that can be used to start flows based on various match criteria.
The following sections describe the most commonly used set of trigger elements.
Text triggers
Text triggers evaluate match criteria against any text input that the user sends via their chat client. Usually the user text will be converted to a say
event that can then be evaluated by text triggers.
catchall
trigger
catchall
triggerThis is the simplest of all triggers and will match when no other triggers could be matched successfully. This is useful to capture any user input that the bot could not "understand".
- catchall
keyword
trigger
keyword
triggerThe keyword trigger will fire when the user text matches exactly with the specified keyword.
- keyword: hi
ignorecase: false
-
hi
: The exact keyword to match with the user text. -
ignorecase
: Ignore the case when matching the user text with the specified keyword.
regex
trigger
regex
triggerThe regex (regular expression) trigger will fire when the user text matches the regex pattern.
- regex: order.*debug
ignorecase: false
-
regex
: The regex (regular expression) pattern to match the user input text against. Meya uses the Python regular expression syntax. -
ignorecase
: Ignore the case when matching the user text.
Button triggers
button_id
trigger
button_id
triggerThis defines a trigger that will match against the specified button ID, and will be evaluated against all button.click
events the user generates.
- button_id: agent_escalation
when: true
-
button_id
: The static button ID to match against. Use this button ID in thebutton_id
field in the relevant quick replies and buttons. -
when
: Optionally set the criteria when this trigger should evaluate. The default istrue
and will always evaluate.
Usually the app runtime will automatically generate button IDs and activate dynamic triggers for these button IDs, however, you can optionally set the button_id
field for a quick reply or button. In this case no dynamic trigger is activated and you will need to explicitly create a button_id
trigger to handle the button.click
event.
These types of buttons are also referred to as static buttons, because you need to explicitly define the button_id
fields and triggers.
The static button trigger can be very useful for handling "external" button clicks, for example, you can use the Meya Orb Web client on your web page and then program an HTML button on your web page to send a static button click event to the Meya Orb Web client and trigger a flow. The demo-app
has an example of a HTML button click that triggers a static button.
Dynamic triggers
Dynamic triggers are triggers that get defined by component elements and is the main mechanism that allows a component to capture and react upon a user's input. When a dynamic trigger get's activated, the flow will pause and wait for a user action.
As a bot developer you generally would not need to define dynamic triggers when using the standard Meya SDK input components, however, if you want to create your own custom input components then you will need to create dynamic triggers to handle and validate user input.
The simplest input component that defines a dynamic trigger is the ask
component. This component sends a message to the user and then waits for the user's input. Here is an outline of what happens when an ask
component is executed:
- The component sends a
say
event to the user. - The component activates a dynamic
catchall
trigger. - The user types a response.
- The bot first evaluates any dynamic triggers.
- The dynamic
catchall
trigger matches and then executes trigger's action.
5.1. In this case the dynamic trigger's action will be anext
component.
5.2. In more complex input components (e.g. email.address.ask) the component could optionally perform input validation. In that case the trigger's action would be to send the user an error message and re-activate the dynamic trigger if the input validation failed. - The dynamic trigger will save the user's input to the
(@ flow.result )
flow scope variable. - The flow will proceed to the next step.
Components that create dynamic triggers
- text.ask
- text.regex_input
- email.address.ask
- email.address.ask.form
- file.ask
- image.ask
- button.ask
- tile.ask
- tile.checkbox
- tile.choice
- tile.rating
- widget.page
Quick reply dynamic triggers
The usual behaviour of a component is to continue the flow since it is “output-only”. The say and image components are two examples.
However, output-only components can have dynamic triggers added to them by defining quick replies that use the action
, result
, or data
fields. These fields change the quick reply’s inferred type to action
or flow_next
, which create dynamic triggers and cause the component to wait for the user’s response before continuing.
NLU triggers
These use NLU (Natural Language Understanding) providers such as Dialogflow, Wit.ai or Luis to match intents based off the user's text input. NLU triggers are very useful for resolving the user's intent from complex sentences.
For example, if a user types:
User input: Show me all the coffee shops in downtown Toronto
You can train an NLU model to classify this type of sentence to be a coffee_search
intent, as well as extract the word Toronto as a city entity.
dialogflow
trigger
dialogflow
triggerThe google.dialogflow
trigger will call your Dialogflow agent's "detect intent" API with the user's input text, and will match if the intent confidence threshold is reached.
If the confidence threshold is reached the intent is evaluated against the specified intent
or intent_regex
. If these fields aren't provided the flow will run and allow the flow to evaluate the intent set in (@ flow.result )
or check the detailed Dialogflow response set in (@ flow. dialogflow_response )
.
- expect: dialogflow
integration: integration.dialogflow
input_context: faq
intent_regex: faq_*.
See the Dialogflow Triggers page for more details and examples.
wit
trigger
wit
triggerThe facebook.wit
trigger will call your Wit model's "message meaning" API with the user's input text, and will match if the intent confidence threshold is reached.
- expect: wit
integration: integration.wit
intent_regex: weather
See the Wit Triggers page for more details and examples.
Trigger precedence
Meya does not restrict you from defining the same type of trigger multiple times, in this case it is useful to understand the order in which conflicting triggers will be processed.
Trigger precedence it determined as follows:
- Ordered alphabetically by the flow file name.
a. For example the triggers in flow filerace-a.yaml
will be processed beforerace-b.yaml
- The order in which the triggers are defined in a flow's
triggers
section.
Here is an example of two flow files that define conflicting keyword
triggers.
triggers:
- keyword: race
action:
jump: race-1
- keyword: race
action:
jump: race-2
steps:
- (race-1)
- say: race-a-1
- end
- (race-2)
- say: race-a-2
- end
triggers:
- keyword: race
steps:
- say: race-b
When entering the keyword race
the output will always be race-a-1
:
flow/race-a.yaml
is processed first.- The first trigger that jumps to
race-1
will be processed first.
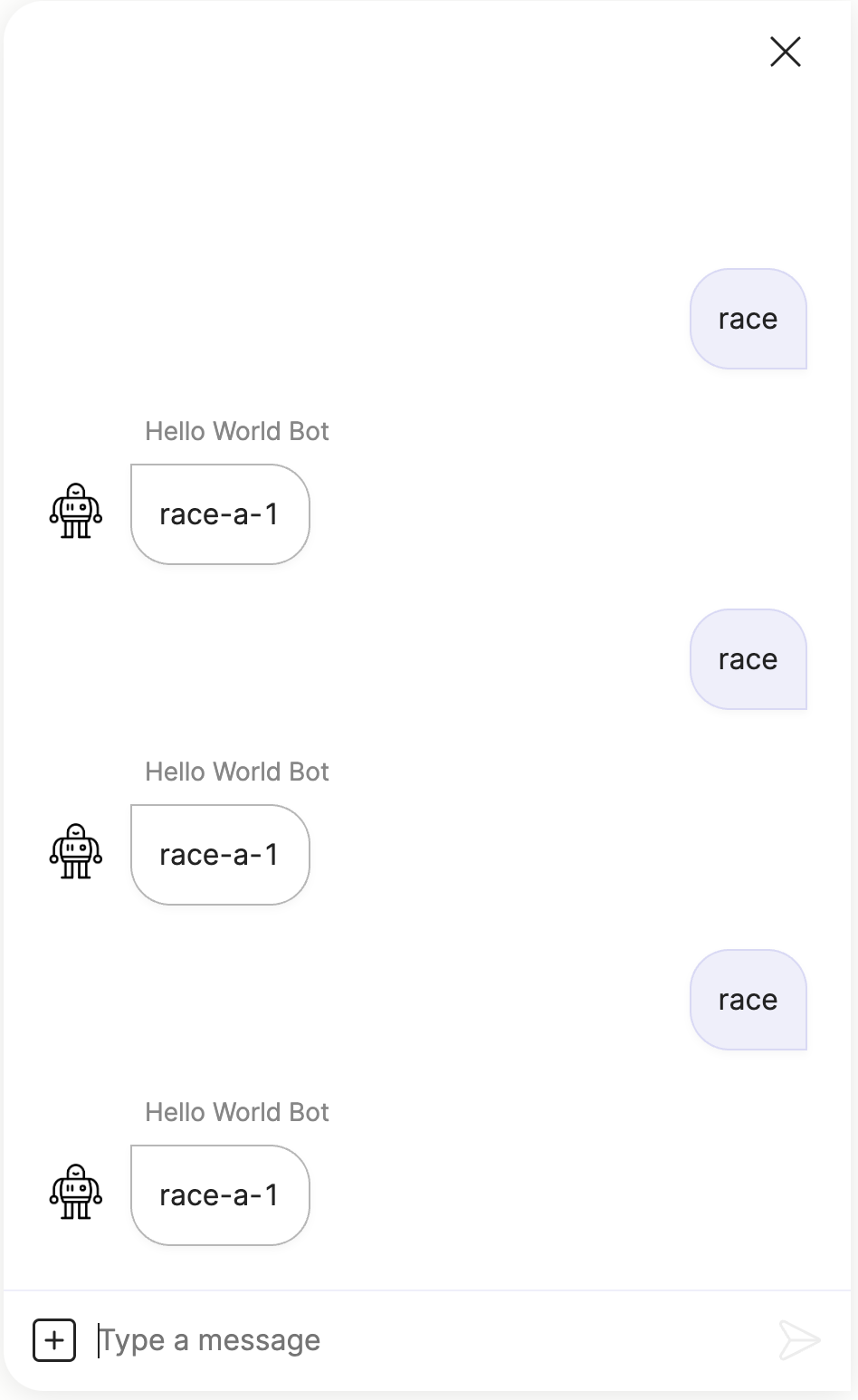
If you change the file name from flows/race-a.yaml
to flows/race-z.yaml
, then the output will always be race-b
:
flow/race-b.yaml
is processed first.- There is only one trigger defined in
flow/race-b.yaml
.
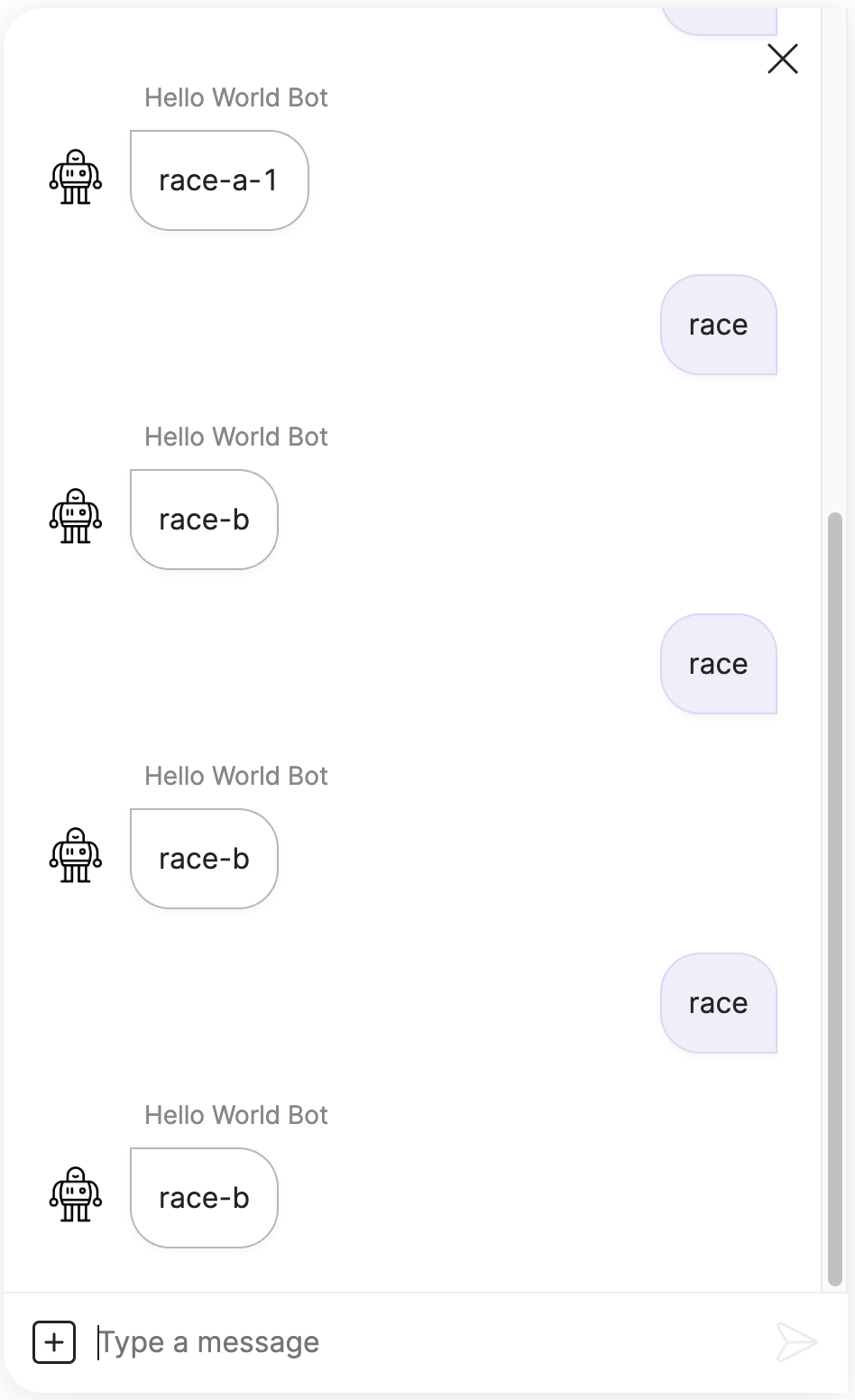
If you change the file name back from flows/race-z.yaml
to flows/race-a.yaml
, but you switch the jump action of the first trigger to be race-2
, then the output will always be race-a-2
.
triggers:
- keyword: race
action:
jump: race-2
- keyword: race
action:
jump: race-1
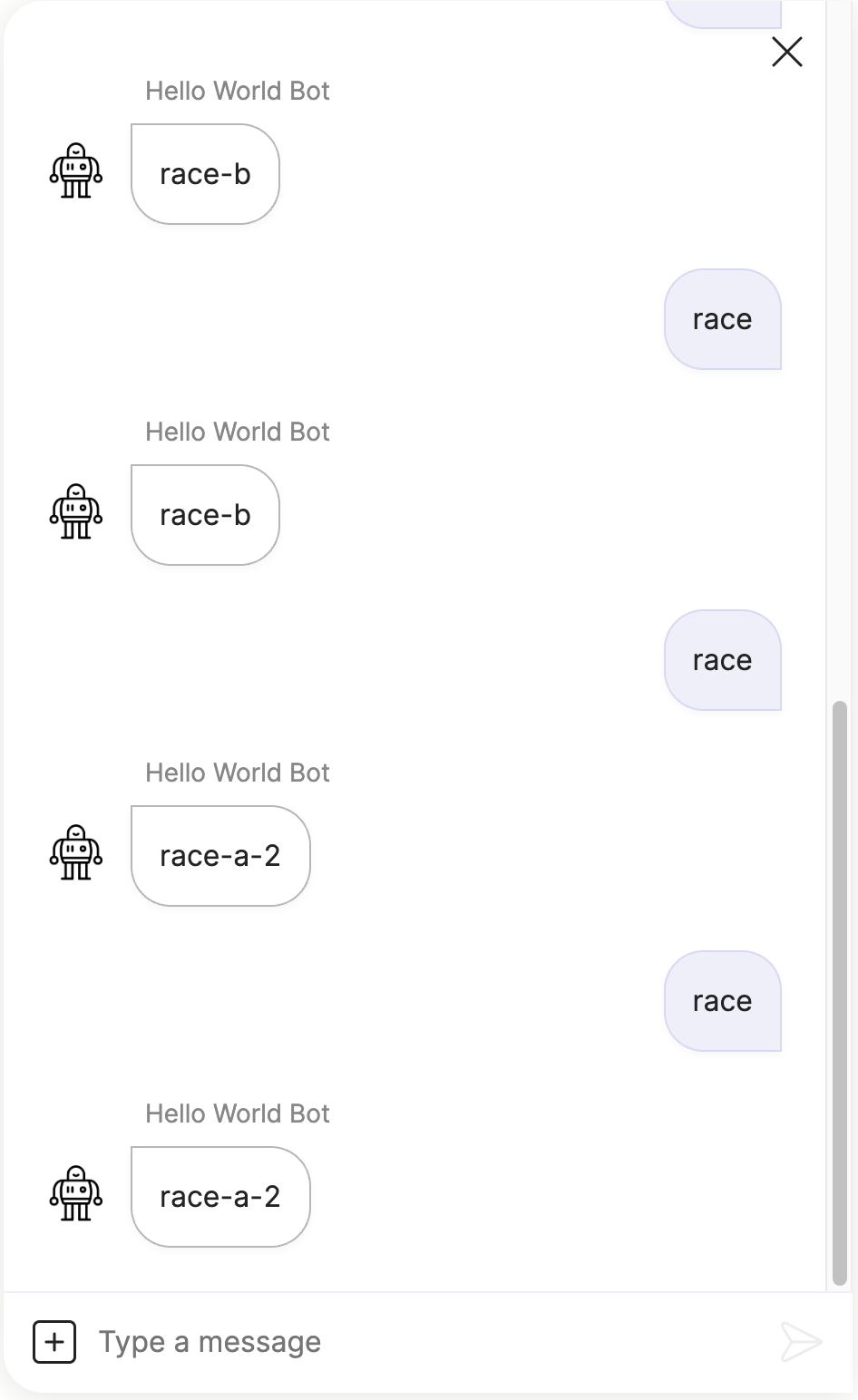
Updated over 1 year ago