Components
A unit of processing within a flow step
Components are elements that are run at each step in a flow. Components are the "work horses" of BFML, and is where input entries are processed and new output entries are generated.
A component consists of the following:
- The component fields that you specify when you define a component in a flow step. (This is also known as the component spec, or in other words, the component's configuration.)
- New entries that are generated by the component.
Below is an example of a simple, and most used, component:
- say: Hello world
quick_replies:
- text: Check the weather
action:
jump: check_weather
- text: Check stocks
action:
jump: check_stocks
From the above snippet you can see that the component step is defined using a set of component fields, in this case the say
field and the quick_replies
field. When defining these fields, the BFML interpreter will resolve the set of defined fields to a concrete Python class that contains the component implementation.
In this case the say
field is known as a signature field, and will resolve to the SayComponent
class which simply returns a text.say event containing the text specified in the say
field.
In more technical terms, with BFML you are declaratively defining the configuration of each Python component class at each flow step that instructs the app runtime to load and start the Python component with the specific configuration.
Reference documentation
The Meya SDK provides many different components that can be used to do many different things such as control the flow execution, call external APIs, render advanced user interface event entries, store and transform data, etc.
The following sections describe some of the most commonly used component elements.
Component reference paths
Generally a component is identified by its signature fields and won't require you to use it's reference path. However, if you are creating your own custom components, then you will need to reference the component using it's reference path.
A component's reference path is simply the component's file path where the slashes /
are replaced with dots .
, and the file extension is dropped.
(If you're familiar with Python, a component reference path is similar to a Python file's module path.)
Here are some example reference paths:
component/get_order.py
becomes:component.get_order
component/db/queries/select_rows_query.py
becomes:component.db.queries.select_rows_query
To use the component reference path in a component spec, you need to set the type
field of the component spec. Here is an example:
- type: component.get_order
order_id: 12345
retry_count: 3
Component spec
A component element's component spec is the set of fields that can be defined when referencing a component in a flow step. Each field in the component spec maps to a concrete field in the component's Python class, where the field's name, data type and default values are defined.
Signature fields
Certain fields of a component can be marked as a signature field, which tells the BFML interpreter how to reference the component in BFML. Basically, this is syntactic sugar that allows to use fields to reference components instead of always providing the type
field and the component reference path.
For example, the text.say is usually references by just using the say
signature field:
- say: Hello world!
Instead of using the type
field and component reference path:
- type: meya.text.component.say
say: Hello world!
Signature fields are defined by setting signature=True
on the component class's field definition:
...
@dataclass
class SayComponent(InteractiveComponent):
say: Optional[str] = element_field(
signature=True, help="Text to send to the user"
)
...
Output components
Output components enable you to send user interface events to the user, for example a text string, an image or set of tile cards. Using output components is the main mechanism by which information is presented to the user across integrations.
Here are some examples of common output components.
say
component
say
componentThe text.say is the most used and simplest output component in the Meya SDK. It simply takes a text string and creates a text.say event that then gets displayed to the user.
- say: Hello world!
say
: This is the signature field and defines the text string will be displayed to the user.
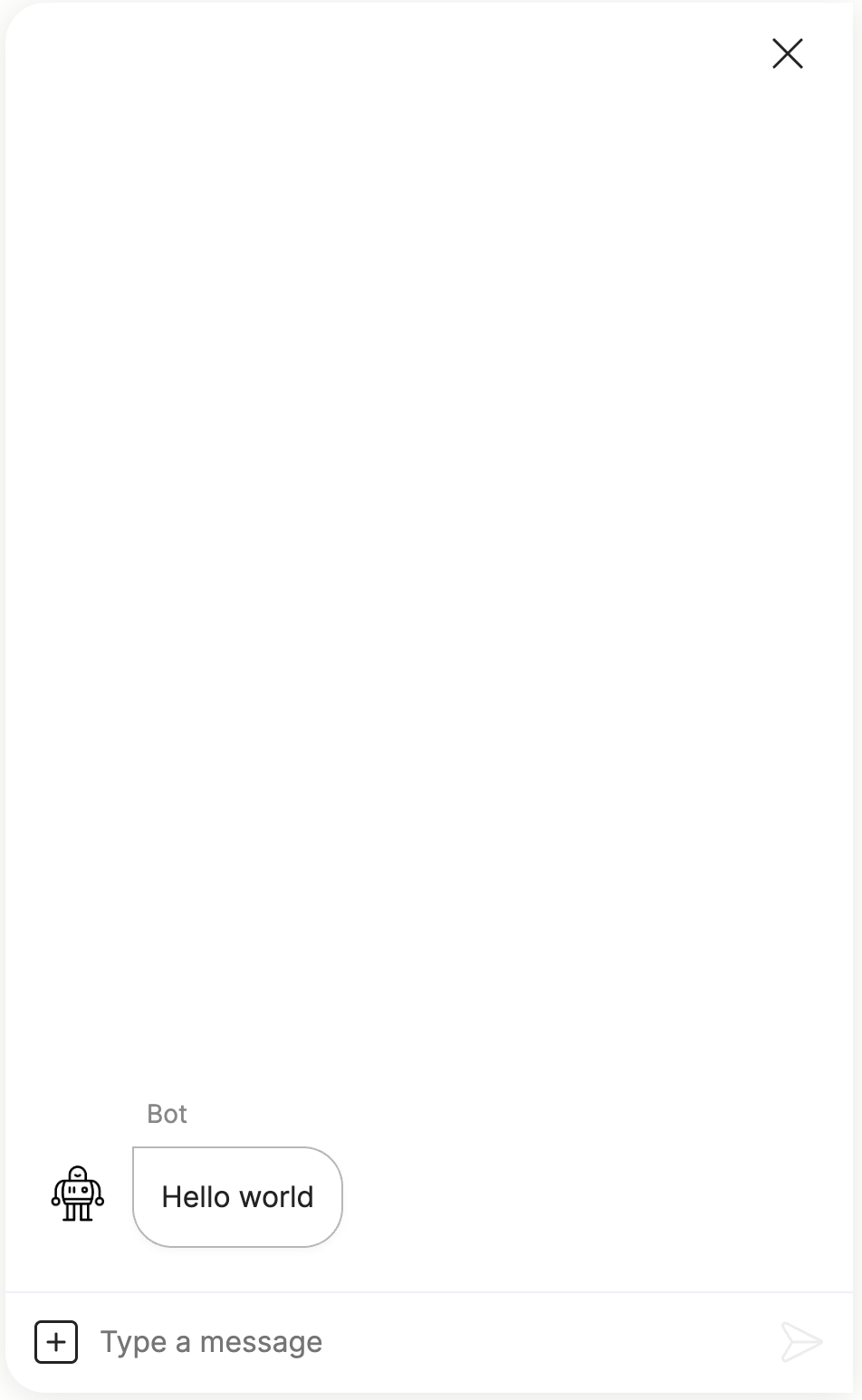
image
component
image
componentThe image component displays an image to the user.
- image: https://images.unsplash.com/photo-1476610182048-b716b8518aae?ixlib=rb-1.2.1&q=85&fm=jpg&crop=entropy&cs=srgb&w=1000
image
: The signature field and defines the URL of the image to be displayed to the user.
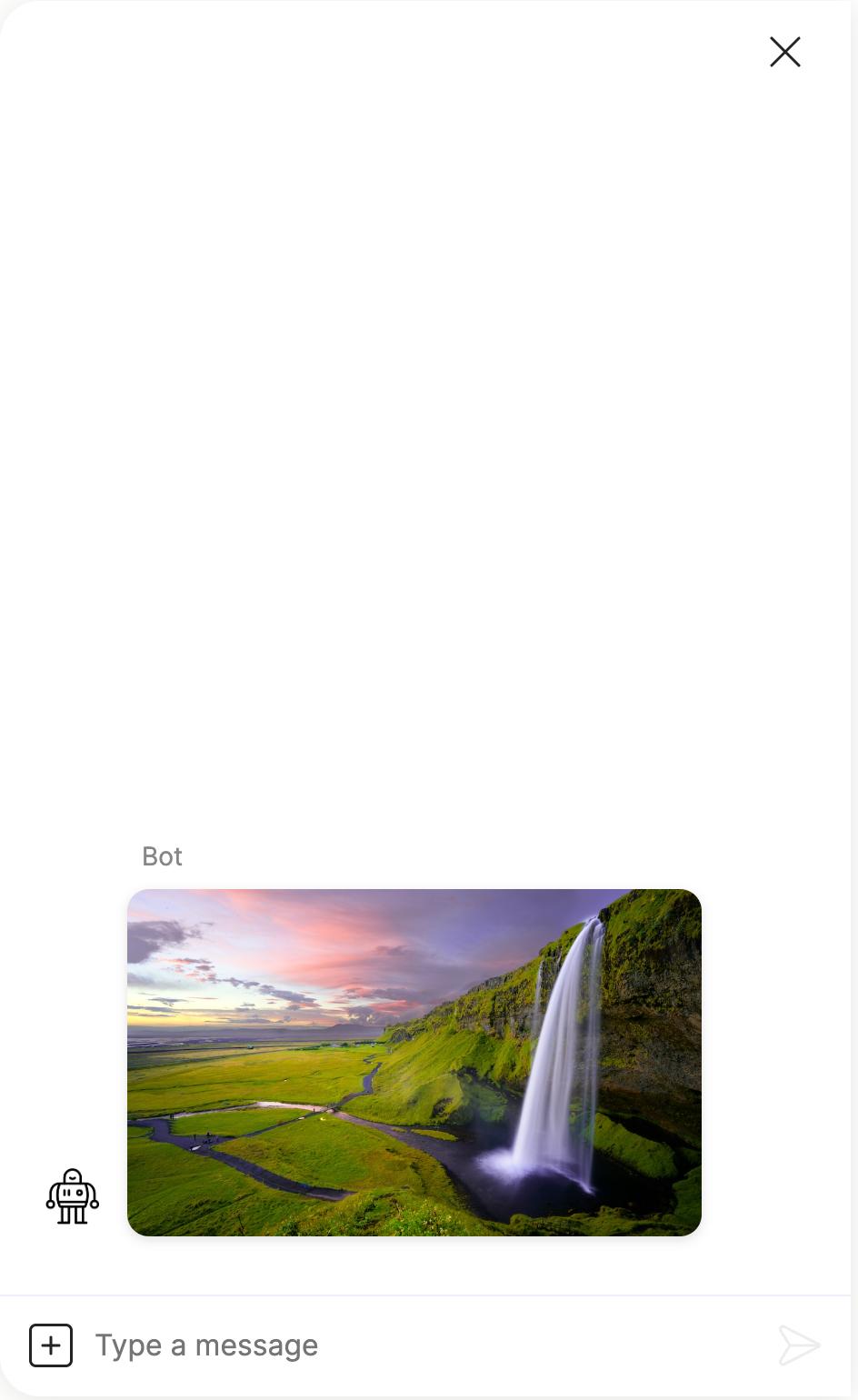
status
component
status
componentThis displays a simple status message to the user.
- status: Bot status message!
status
: The signature fields and defines the status message to be displayed to the user.
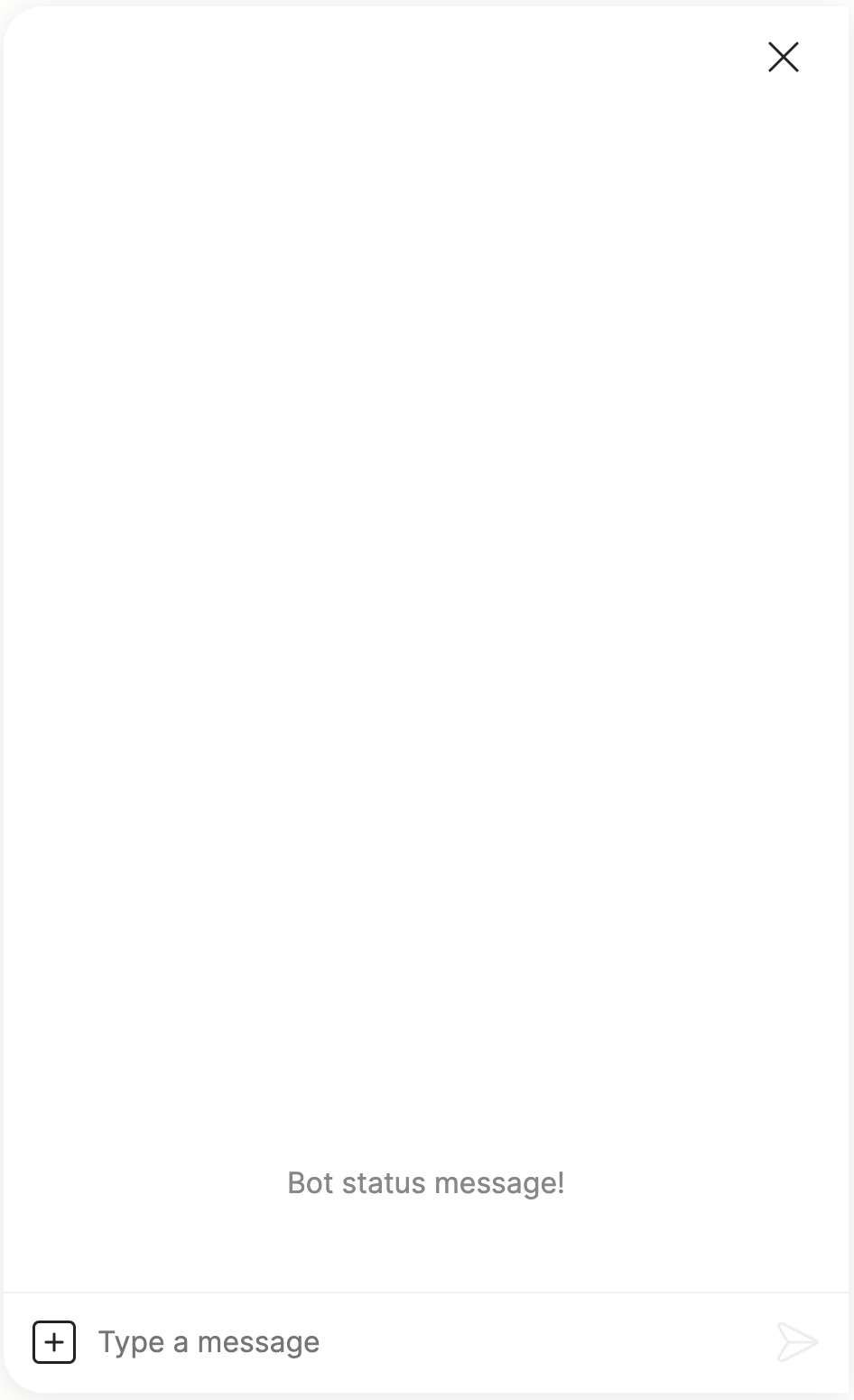
tiles
component
tiles
componentThis displays a row of tiles that the user can browse through. Note that the tiles
component can also be configured to work as an input component, where the user can select a tile or click a button within a tile.
- tiles:
- title: Pixel 4 XL Black
image:
url: https://lh3.googleusercontent.com/1cAx0YybaOQTBwtk7gC4Ndef4w__Lg0MT-TsY7O5ycMnEEVF0l3YTtyrEGYhWNINzw=rw-w1520
result: pixel-4-xl-black
- title: Pixel 4 XL White
image:
url: https://lh3.googleusercontent.com/qFd_GwwRdmU6bePdrwf-R6mYhTtFgSJw4do4JGXzrJvnUi0qgUsBFp1L0PIrCllZzd0j=rw-w1520
result: pixel-4-xl-white
tiles
: A list of tile definitions that allow you to set fields such as the title, image, description, buttons etc.
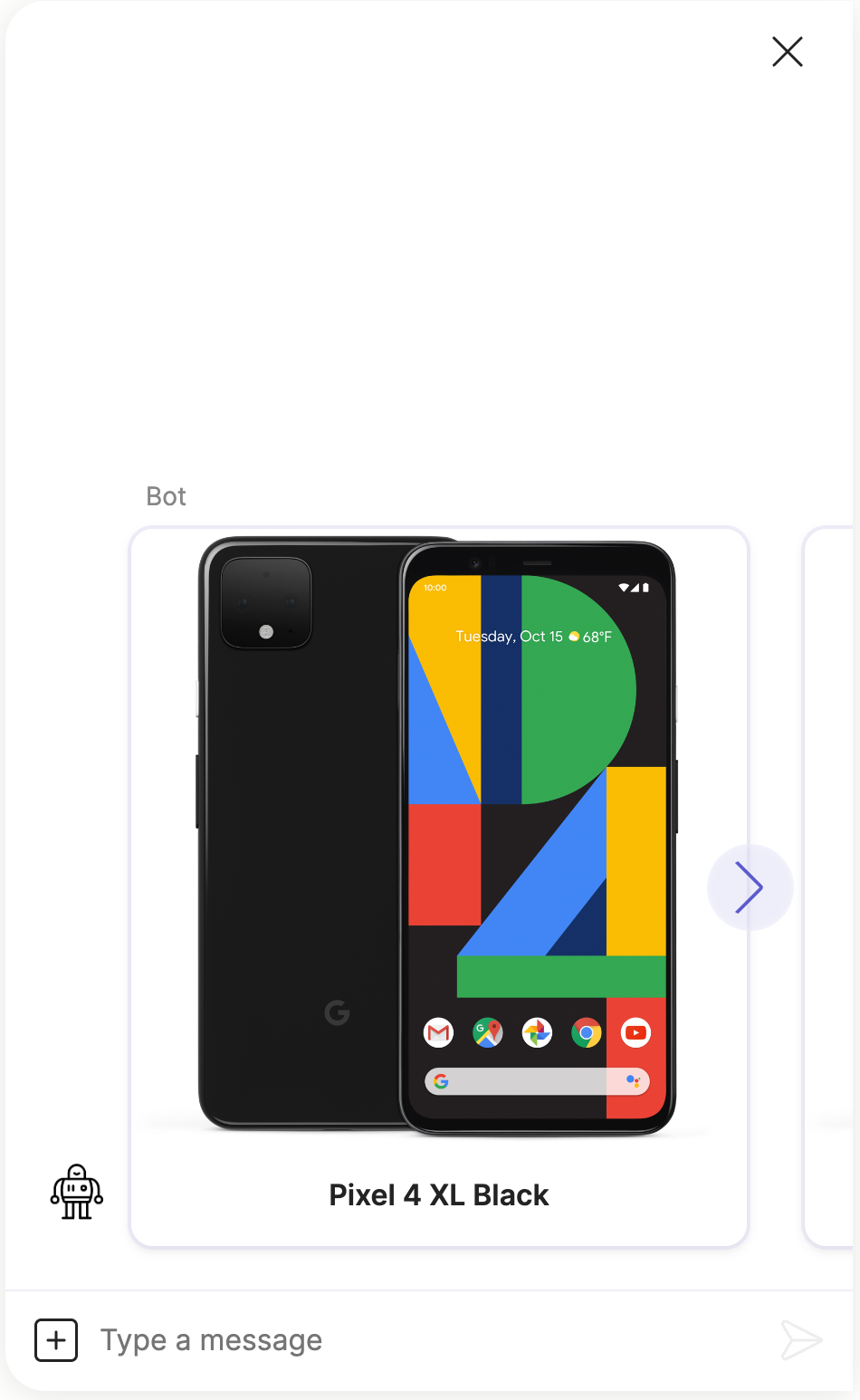
Please check the demo-app
's tile flows for more advanced usages of the tile
component.
Input components
Input components are a special class of components that:
- pause flow execution,
- waits for a user's input,
- validates the user's input, and
- captures the user's input into flow scope.
This is the main mechanism that allows a bot to capture and process a user's input from an integration. Also check how dynamic triggers are used to enable the bot to wait for user input events.
Here are some examples of common input components.
ask
component
ask
componentThe text.ask component is the simplest type of input component that display's a text string to the user and waits for input from the user.
- ask: What is your name?
ask
: This is the signature field and defines the text string will be displayed to the user.
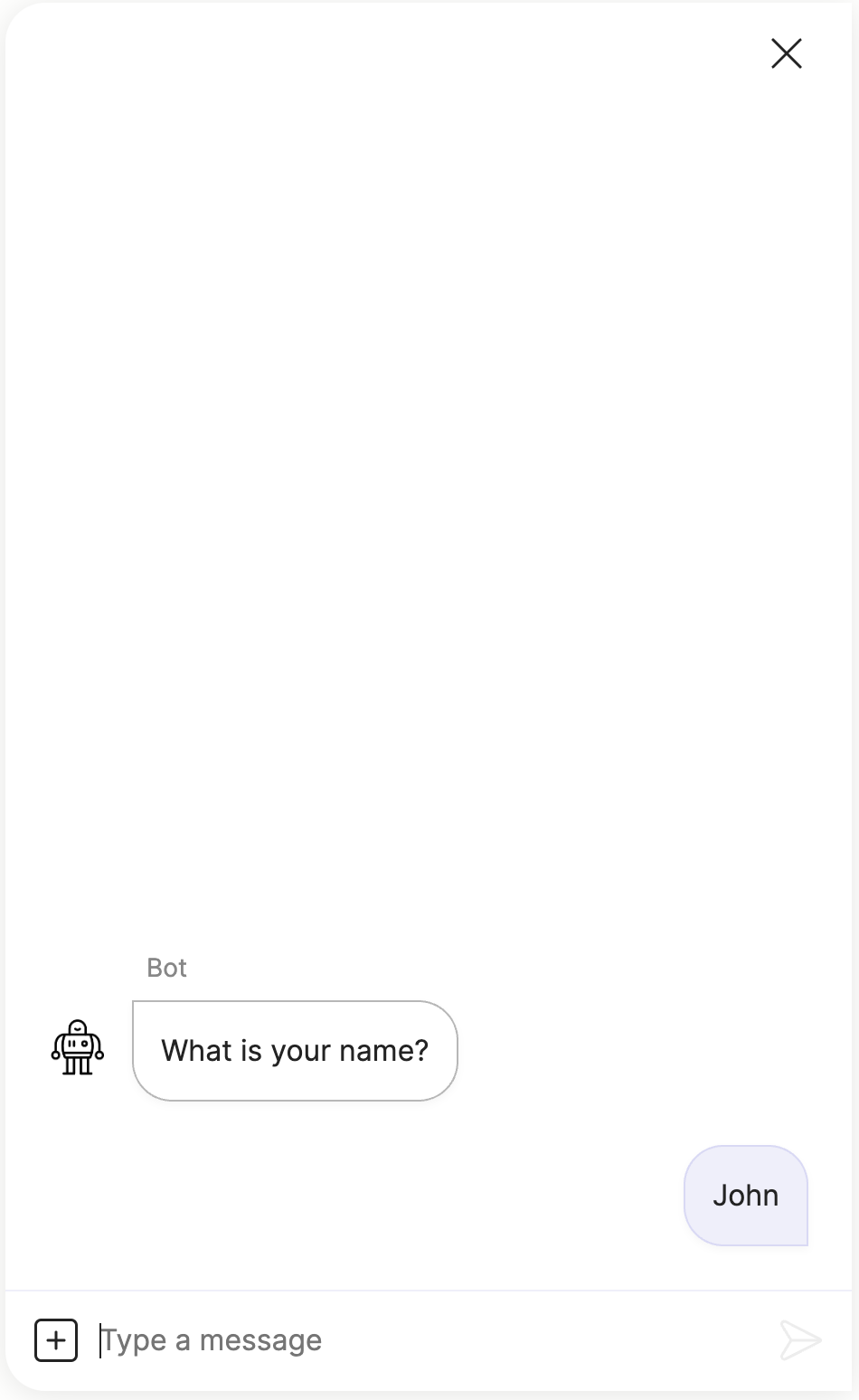
buttons
component
buttons
componentThis component will display a group of buttons that they user can select from.
- buttons:
- text: Option 1
result:
key: 1
- text: Option 2
result:
- two
- two
- text: Text 3
- text: Link 4
url: https://example.org/?n=5
- text: Static 5
button_id: _static_button
context:
product: marmalade
- text: Menu 6
menu:
- text: Result 10
result: 10
- text: Result 11
result: 11
- text: Result 20
result: 20
- text: Disabled
disabled: true
buttons
: A list of tile definitions that allow you to set button fields such as the text, button_id, url, disabled etc.
Please check the demo-app
's button flows for more advanced usages of the buttons
component.
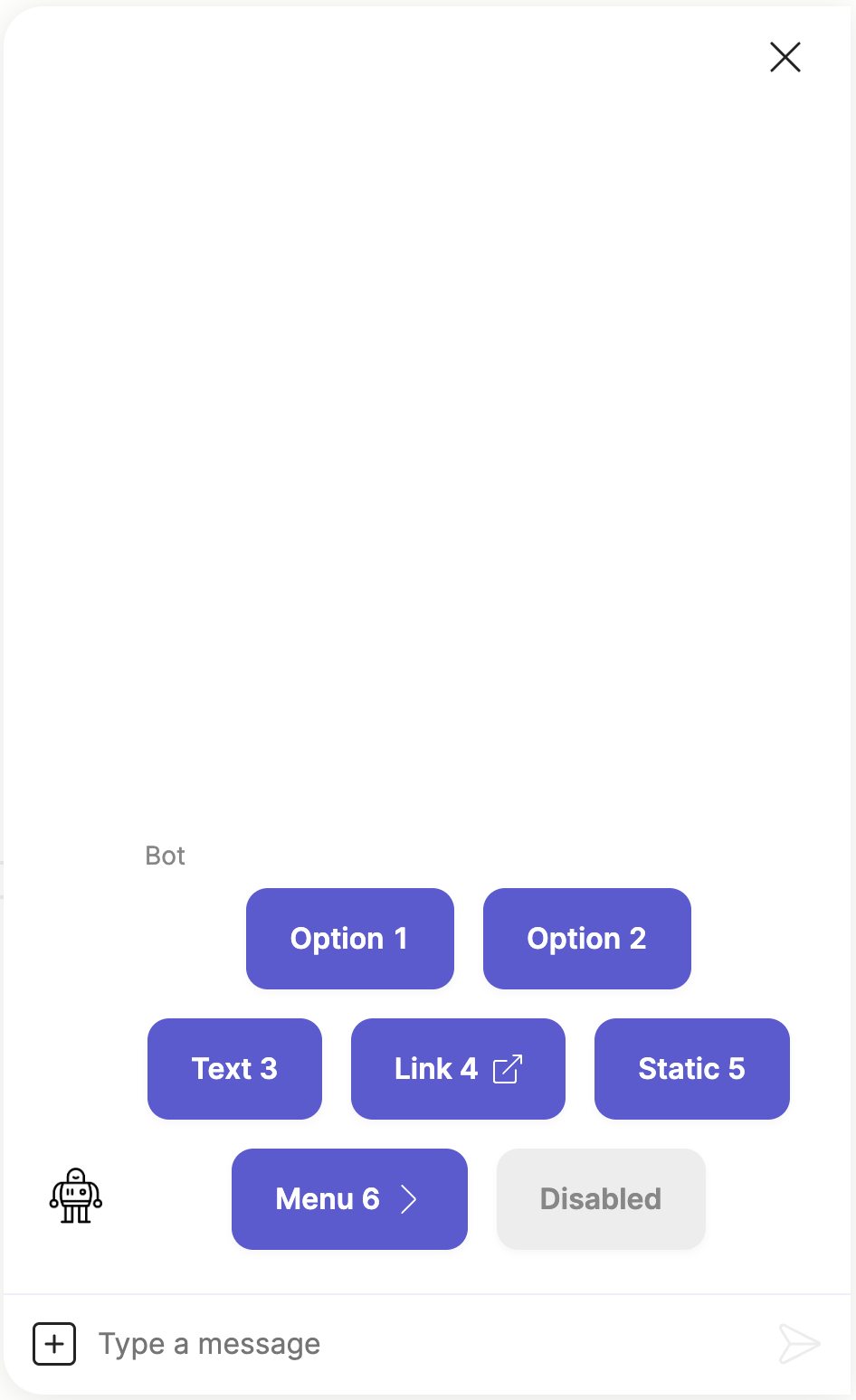
Utility components
Utility components are components that do not wait for input nor display any output, but rather they perform some internal action such as saving data to a data scope, or calling an external API, or branching the flow.
flow_set
component
flow_set
componentThis component stores data to the flow scope.
- flow_set:
foo: bar
name: (@ flow.result )
flow_set
: This is the signature field and takes an arbitrary dictionary of keys and values. After the component is called, the defined keys will be available to query using template tags e.g.(@ flow.foo )
and(@ flow.name )
.
Note, if you are only setting a single value from (@ flow.result )
then you can just specify the key name for flow_set
.
- flow_set: name
This will automatically store (@ flow.result )
under the name
key.
thread_set
component
thread_set
componentThis component stores data to the thread scope.
- thread_set:
foo: bar
otp: (@ flow.result )
thread_set
: This is the signature field that takes an arbitrary dictionary of keys and values. After the component is called the defined keys will be available to query using template tags e.g.(@ thread.foo )
and(@ thread.otp )
.
Note, if you are only setting a single value from (@ flow.result )
then you can just specify the key name for thread_set
.
- thread_set: otp
This will automatically store (@ flow.result )
under the otp
key.
user_set
component
user_set
componentThis component stores data to the user scope.
- user_set:
foo: bar
email: (@ flow.result )
user_set
: This is the signature field that takes an arbitrary dictionary of keys and values. After the component is called the defined keys will be available to query using template tags e.g.(@ user.foo )
and(@ user.email )
.
Note, if you are only setting a single value from (@ flow.result )
then you can just specify the key name for user_set
.
- user_set: email
This will automatically store (@ flow.result )
under the email
key.
delay
component
delay
componentThis will cause the flow to pause for a predetermined amount of time.
- delay: 5
delay
: This is the signature field that specifies the number of seconds to wait. This can be a decimal number e.g.0.5
.
Note, there is a maximum execution time limit of 35s for a component, so if you set the delay to be larger than 35s the component will raise a timeout error and stop the flow.
Integration components
There are many components that are specific to integrations which give you more fine grained control of the integration from BFML. These components are especially useful when using CSP (Customer Service Platform) integrations where you need to query agent schedules, annotate a ticket/conversation with extra data, or manage the user contact.
Check the integration pages and component reference for the specific component documentation.
Be careful when using integration specific components, especially for messaging integrations such as Facebook, WhatsApp, Telegram etc.If you are using multiple messaging integrations, then a specific messaging integration component might not be compatible with other messaging integrations. This also applies to advanced output components such as the
tiles
component.Check the Compatibility Matrix to see which output components are supported by the various integrations.
Page components
Page components are a special class of components that allow you to display a page containing a set of form widgets when using the Meya Orb Web/Mobile SDK.
Page components allow you to create very flexible input form wizards with validation and advanced flow control.
Check the pages-demo
app for a complete example implementation.
Here is an example of a form wizard implemented using pages and form widgets:
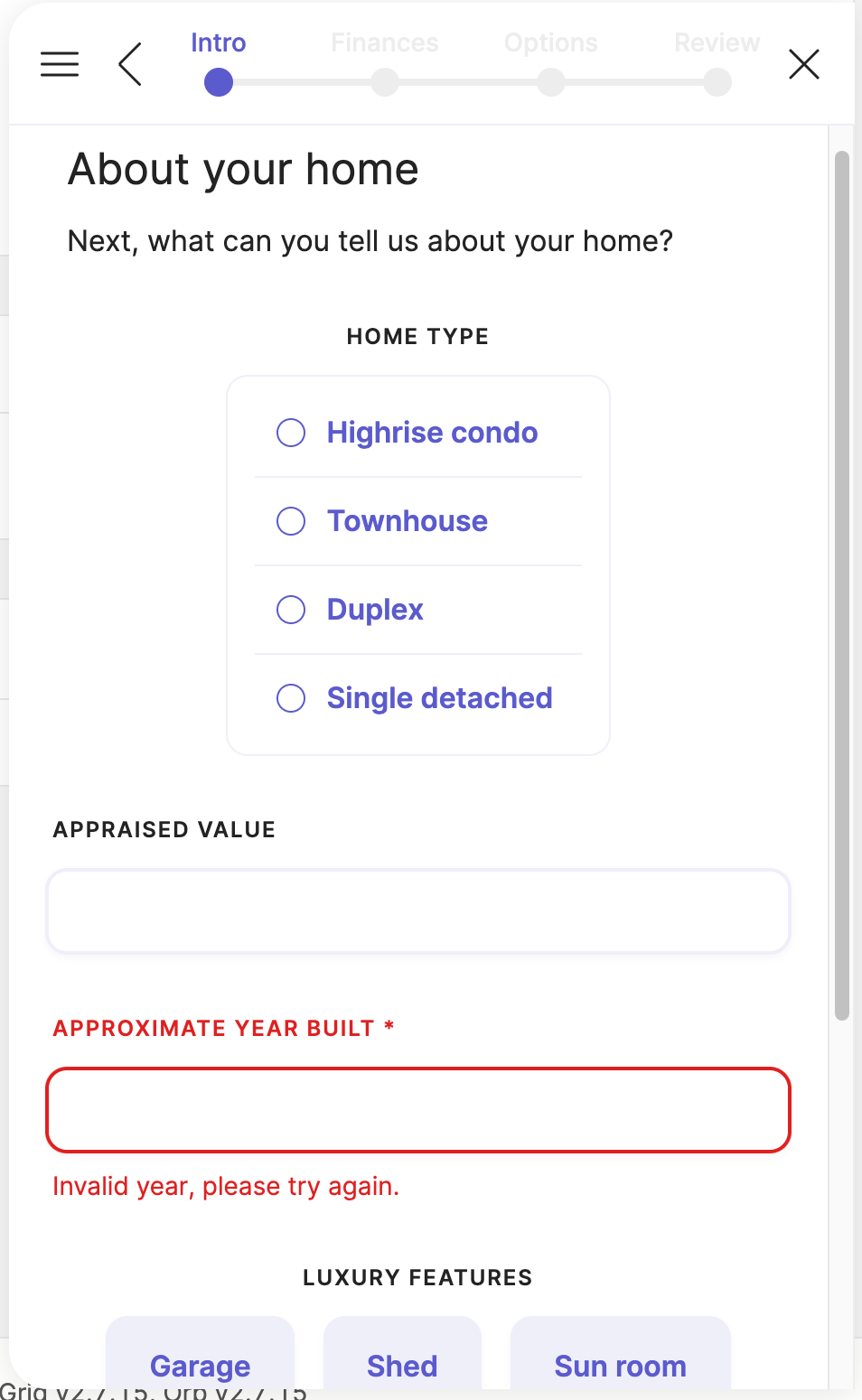
Updated about 1 month ago