Overview
Bot Flow Markup Language
BFML is a proprietary domain specific language (DSL) created by Meya that helps create advanced conversational applications. It's based on YAML and is easy to learn and very powerful.
- BFML is to a conversational app as HTML is to a web app
- Based on YAML
It looks like this...
triggers:
- keyword: hi
steps:
- say: Hello!
Which produces this...
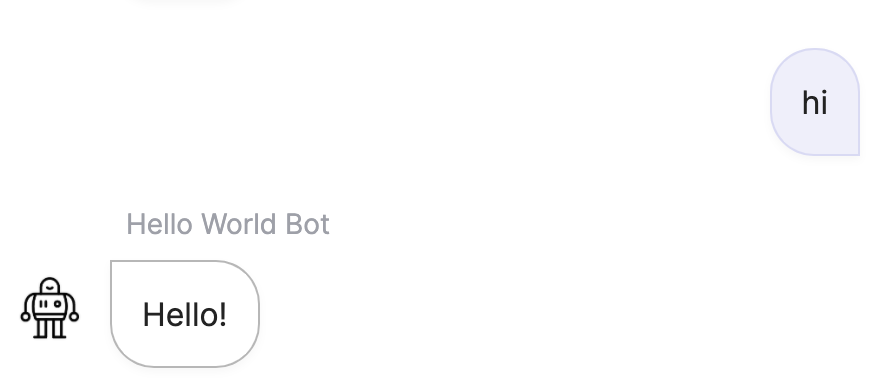
Introduction
BFML Elements
BFML files can contain three types of elements, namely:
-
Bot: A bot element contains a collection of flow elements and trigger elements. These contain all the work flows a bot is programmed to perform e.g. questions & answers flows, knowledge base searchs, authentication flows, purchasing flows, human agent escalation flows etc. In Meya an app can technically contain multiple bot elements, but generally there is only one bot programmed to handle all the work flows.
-
Flow: A flow element contains a list of trigger elements under the
triggers
keyword, and a list of components under thesteps
keyword. Flows are stored in a flow YAML file with the.yaml
file extension. (Using a programming analogy, you can think of a flow element as being the program file, where triggers and components are types event listeners and functions.) -
Trigger: A trigger element listens to certain events and will match on certain criteria. Once a trigger matches, it will execute a flow.
-
Component: Once a flow is started by a matching trigger, the flow runs through a sequence of steps, where each step executes a component element. A component element is a special Python class that is programmed to perform certain actions, this could be calling an external API, storing/mutating some data, or producing more event entries.
-
Integration: An integration element is a special element that listens to requests from external systems as well as events produced by component elements, and then relay these events to trigger elements and external systems. Requests made from or to external systems are usually either HTTP, or Websocket requests.
Have a look at our demo-app source (check out the /flow
folder) for lots of examples of BFML.
Entries
It is very helpful to understand how a bot in a Meya app handles and processes data.
At its core a Meya app is a special form of what we call a data streaming application. In generic terms, this simply means that BFML elements receive data entries from a data stream, processes these entries, and optionally posts new entries to the data stream.
In Meya we refer to these data streams as ledgers, where each piece of data stored in a ledger is called a ledger entry.
As a bot developer you generally do not need to think about ledgers because BFML and the Meya SDK abstracts this away, but it is helpful to understand the different types of ledgers especially, when viewing your app's logs in the Console logs view.
Ledgers
There are 8 different ledgers that each contain a stream of specific types of entries.
http_ledger
http_ledger
This ledger contains all the HTTP request and response payloads your app sends and receives to/from external systems.
ws_ledger
ws_ledger
This ledger contains all the Websocket request and response payloads your app sends and receives to/from external clients. (Currently the only integrations that support Websockets are the Meya Orb Web SDKand Meya Orb Mobile SDK integrations.)
event_ledger
event_ledger
This ledger contains all the UI (user interface) events e.g. user/bot text, user button click, page open, chat open etc. that occurs in a user <-> bot conversation thread.
When you implement your own custom components, triggers or integrations in Python, you will generally be processing and be creating event entries that will be displayed to the user. For example, you can create a tile.ask entry that will display a list of tile cards that can contain images, descriptions, titles and buttons a user can click.
thread_ledger
thread_ledger
This is a special ledger used to store entries that update the app's thread data scope.
In Meya each user <-> bot conversation is stored in a conversation thread, where each thread can contain multiple participants e.g. bot, user, agent. When you create BFML flows you can program the bot to store information relevant to the conversation thread e.g. a list of product ids in the user's shopping cart.
user_ledger
user_ledger
This is a special ledger used to store entries that update the app's user data scope.
In Meya each unique user that interacts with a bot in a Meya app has their own data store. You can then program the bot to store information pertaining to the user in this data store. For example, when you request the user's name and email address, you would usually store this in the user's data store.
The user data scope becomes particularly important when authenticating the user with 3rd party systems.
presence_ledger
presence_ledger
This ledger contains user presence entries such as typing on or device state.
log_ledger
log_ledger
This ledger contains app log information. If your bot throws an error exception, or you would like to print certain debug information as you are creating flows, then these log entries are stored in the log ledger.
bot_ledger
bot_ledger
This ledger contains all the bot flow entries e.g. flow start, component start etc.
The bot flow entries contain the information required to control the bot's flow execution, in other words, which flow step to execute next. Bot flow entries also contain flow scope data as well as the flow call stack when executing nested flows. (This is analogous to a program's program counter, call stack and heap memory in conventional programming languages such as Python.)
Note: as a bot developer, you will not need to create your own flow entries, these are created by the BFML runtime. These entries are, however, very useful when debugging your bot's flow scope data, and flow call stack in the Console logs view.
Updated about 2 months ago