Testing Flows
Programmatically test your flows
You may already be using your app's /status
API endpoint to monitor it's uptime and latency, but did you know you can also use it to test your flows? Here's how.
Triggering a flow
Let's review what the /status
endpoint looks like:
https://grid.meya.ai/gateway/v2/status/<APP_ID>/__status__?user_id=monitor&text=__status__
CachingYou must set
Cache-Control: no-cache
in the header when testing any endpoint to ensure you don't get a cached result.
Replace APP_ID
with your app's actual ID, which can be found on your app's Settings page.
You can leave the user_id
to monitor
. This ensures that your testing will only use a single Meya user, instead of generating a new one every time.
You can fire a flow by changing the value of the text
query parameter. For example, you would use text=_custom
to trigger this custom.yaml
flow:
triggers:
- keyword: _custom
steps:
- say: first step
- flow_set:
x: (@ 2 * 2 * 2 )
- say: second step x=(@ flow.x )
Using keywordsTo guarantee you trigger the right flow, consider adding a
keyword
trigger to each of your flows. Make the keyword something a user is unlikely to say, like_test_welcome_flow
.
This is the Orb simulator output for the above flow:
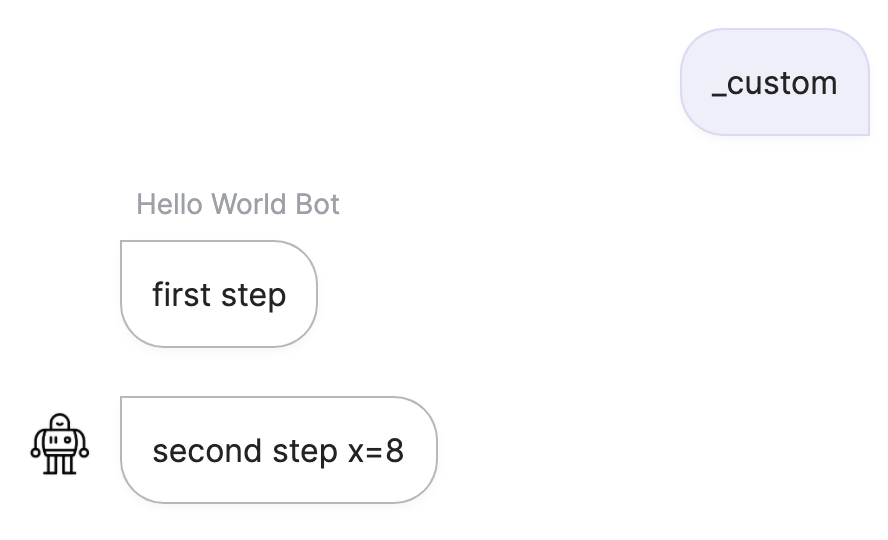
And here's the response you'd get from your API call:
{
"ok": true,
"text": "first step second step x=8",
"time": "2020-09-09 19:42:21.875174"
}
Automated testing
With the /status
endpoint, you can write a script to run a series of tests of your flows and compare the response text with the expected text. Run the script as a cron job and now you have ongoing automated testing of your flows!
Here's a sample Python script that stores the test phrases and their expected responses in a dictionary:
import requests
URL = "https://grid.meya.ai/gateway/v2/status/APP_ID/__status__?user_id=monitor&text="
HEADERS = {
"Cache-Control": "no-cache",
}
tests = {
"_custom": "first step second step x=8",
"hi": "Hi! What would you like to do?",
}
def main():
for test in tests:
res = requests.get(f"{URL}{test}", headers=HEADERS)
if res.status_code != 200:
print(f"Test <{test}> failed! Status code: {res.status_code}")
continue
data = res.json()
actual = data.get("text")
expected = tests[test]
if actual != expected:
print(f"Test <{test}> failed! Expected: {expected}; Actual: {actual}")
else:
print(f"Test <{test}> passed.")
if __name__ == "__main__":
main()
Automated browser testing
Another option for testing your app is to use a tool like Selenium, which allows you to automate browser interactions. With Selenium, you can record your interactions with the browser and replay them later.
The image below shows a basic example. When the play button is clicked, it opens a new browser window, opens the Orb chat, and sends the word hi
to the app.
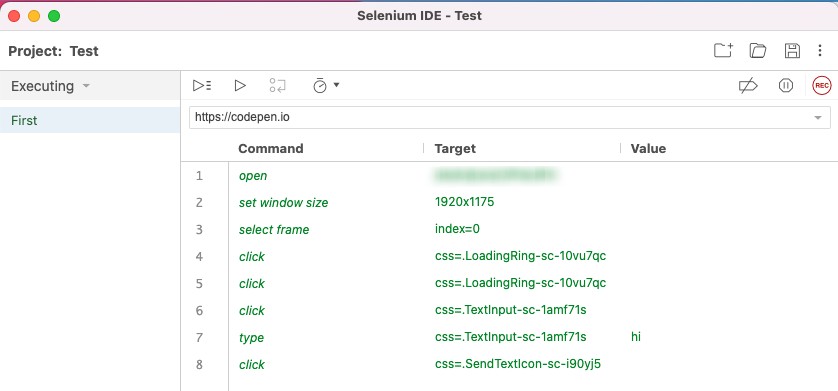
A simple test in Selenium
Updated about 2 months ago