Agent-initiated conversations (Orb)
Agent-initiated conversations from Zendesk Support to Meya Orb
This is an advanced use-case specific to Zendesk Support and Meya Orb web/mobile clients when you want to start an in-app support conversation from the agent (i.e. a proactive message).
Client authentication
For this use-case you need to ensure that the Meya Orb web/mobile SDK is authenticated using the user's email address.
Note lines 8
and 9
which contains the user email address.
<script type="text/javascript">
window.orbConfig = {
connectionOptions: {
gridUrl: "https://grid.meya.ai",
appId: "app-1234...",
integrationId: "integration.orb",
connect: false,
userId: "[email protected]",
threadId: "[email protected]",
},
windowApi: true,
};
(function(){
var script = document.createElement("script");
script.type = "text/javascript";
script.async = true;
script.src = "https://cdn.meya.ai/v2/orb.js";
document.body.appendChild(script);
var fontStyleSheet = document.createElement("link");
fontStyleSheet.rel = "stylesheet";
fontStyleSheet.href = "https://cdn.meya.ai/font/inter.css";
document.body.appendChild(fontStyleSheet);
})();
</script>
Here is an example for the Meya Orb Mobile SDK in iOS.
...
let connectionOptions = OrbConnectionOptions(
gridUrl: "https://grid.meya.ai",
appId: "app-1234...",
integrationId: "integration.mobile",
userId: "[email protected]",
threadId: "[email protected]"
)
...
Also check the orb-demo-ios
for other connection options.
Here is an example for the Meya Orb Mobile SDK in Android:
...
String gridUrl = "https://grid.meya.ai";
String appId = "app-1234...";
String integrationId = "integration.mobile";
OrbConnectionOptions connectionOptions = new OrbConnectionOptions(
gridUrl,
appId,
integrationId,
);
connectionOptions.userId = "[email protected]";
connectionOptions.threadId = "[email protected]";
...
Also check the orb-demo-android
for other connection options.
Add the unhandled ticket flow
triggers:
- type: meya.zendesk.support.trigger.ticket.unhandled
steps:
# Try to find the ZDS Meya user link
- flow_set:
zds_integration_user_id: (@ flow.requester.id | string )
- user_try_lookup: (@ flow.zds_integration_user_id )
integration: integration.zendesk.support
- flow_set: user_id
- if: (@ flow.user_id )
then: next
else:
jump: orb_find_by_app_user_id
# Try to find the Orb Meya user link
- type: user_try_reverse_lookup
user_id: (@ flow.user_id )
integration: integration.orb
- flow_set: orb_integration_user_id
- if: (@ flow.orb_integration_user_id )
then:
jump: orb_user_ready
else: next
# Try to find the Orb user via ZDS app user ID (require a result)
- (orb_find_by_app_user_id)
- flow_set:
orb_integration_user_id: (@ flow.requester.email )
- user_try_lookup: (@ flow.orb_integration_user_id )
integration: integration.orb
- flow_set: user_id
- if: (@ flow.user_id )
then:
jump: orb_user_link
else: next
# Identify the Meya Orb user
- user_identify: (@ flow.orb_integration_user_id )
integration: integration.orb
- flow_set: user_id
# Link the Meya user to ZDS
- (orb_user_link)
- user_link: (@ flow.zds_integration_user_id )
user_id: (@ flow.user_id )
integration: integration.zendesk.support
# Try to find the Orb thread link
- (orb_user_ready)
- flow_set:
orb_integration_thread_id: "(@ flow.requester.email )/(@ flow.requester.email )"
- thread_try_lookup: (@ flow.orb_integration_thread_id )
integration: integration.orb
- if: (@ flow.result )
then: next
else:
jump: orb_thread_link
# Use the existing Orb thread
- flow_set: thread_id
- jump: orb_thread_ready
# Link the new thread to Orb
- (orb_thread_link)
- flow_set:
thread_id: (@ thread.id )
- thread_link: (@ flow.orb_integration_thread_id )
thread_id: (@ flow.thread_id )
integration: integration.orb
# Set the new thread primary user
- thread_set:
primary_user_id: (@ flow.user_id )
thread_id: (@ flow.thread_id )
# Link the Meya thread to ZDS
- (orb_thread_ready)
- thread_link: (@ flow.ticket.id | string )
thread_id: (@ flow.thread_id )
integration: integration.zendesk.support
# Redo ZDS RX
- type: meya.zendesk.support.component.ticket.rx
integration: integration.zendesk.support
thread_id: (@ flow.thread_id )
ticket: (@ flow.ticket )
current_user: (@ flow.current_user )
Update your Zendesk Support integration settings
You need to enable the support for unhandled tickets. Those are Zendesk Support tickets that are not linked to any users in Meya (yet). See this example code.
You can specify alternate value for ticket.tags
other than orb_send
in order to trigger the unhandled flow based on your agent workflow.
type: meya.zendesk.support.integration
subdomain: (@ vault.zendesk.support.subdomain )
bot_agent_email: (@ vault.zendesk.support.bot_agent_email )
bot_agent_api_token: (@ vault.zendesk.support.bot_agent_api_token )
target_password: (@ vault.zendesk.support.target_password )
markdown: true
extract_html_links: false
unlink_ticket_status: [closed, solved]
include_text_with_media: true
filter:
rx_unhandled_ticket: >
ticket.tags:orb_send
AND ticket.status:(new OR open OR pending)
AND current_user.role:(NOT end-user)
Creating an agent-initiated ticket
- Create/select the user you want to initiate a conversation with using their email address.
- Click the + New Ticket button.
- Important: add the
orb_send
tag. (If you don't add the tag then the Meya integration will ignore the ticket webhook.) - Specify a Subject. (This is ignored in Orb.)
- Type a message.
Using custom user ID and thread ID
Using the user's email address is the simplest way to implement agent-initiated conversations from Zendesk Support, this is because the user's email address is a primary field in Zendesk Support.
However, you may prefer to use an alternative ID than the user's email address. In this case you will need to add custom user fields to track these IDs.
1. Add custom user fields in Zendesk Support
Add two custom user fields in the Zendesk Admin section:
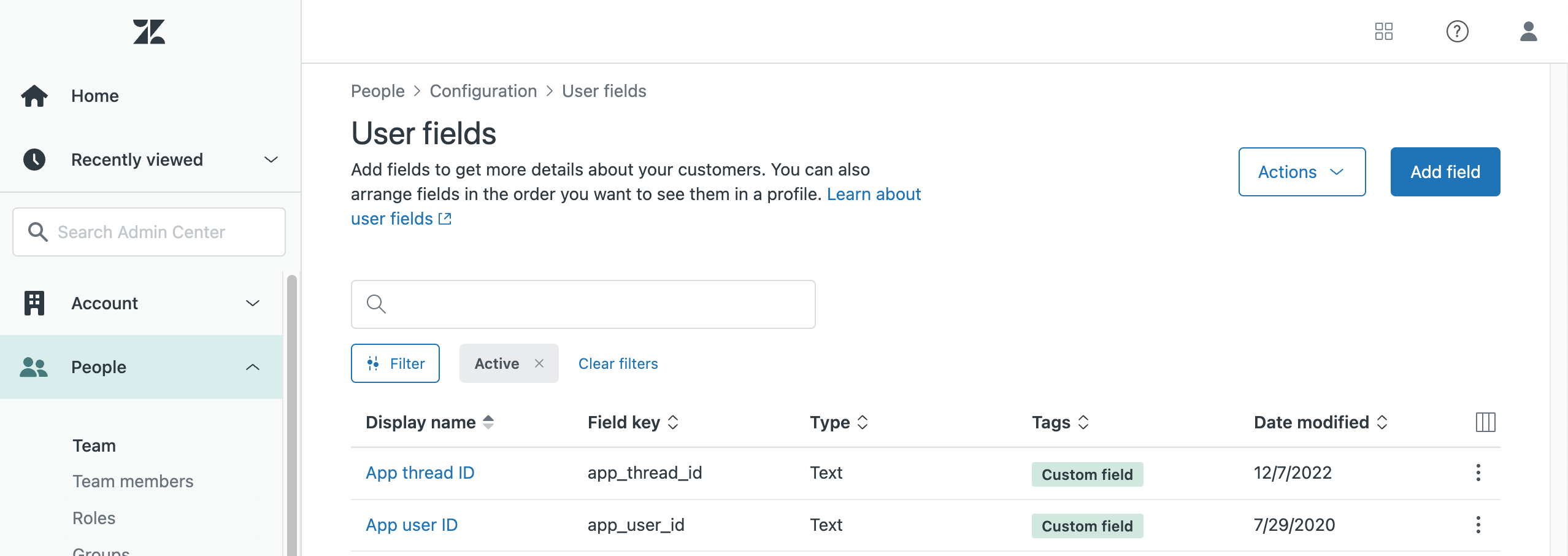
In this case we specified the field keys to be app_user_id
and app_thread_id
, but you can set this to something that makes sense to you. (Just remember these field keys are referenced in the unhandled ticket flow.)
2. Update your unhandled ticket flow
All we need to do is change the section of the flow where the orb_integration_thread_id
is set:
...
# Try to find the Orb thread link
- (orb_user_ready)
- flow_set:
orb_integration_thread_id: "(@ flow.requester.user_fields.app_user_id )/(@ flow.requester.user_fields.app_thread_id )"
...
3. Update you Mobile Orb connection options
Update your Meya Orb connection options to use your custom user ID and thread ID.
Note, you can make user ID and thread ID have the same value.
connectionOptions: {
gridUrl: "https://grid.meya.ai",
appId: "app-1234...",
integrationId: "integration.orb",
connect: false,
userId: "1234",
threadId: "1234",
},
The same will apply for the Meya Orb Mobile SDK.
4. Update the user in Zendesk Support
- Create/select the user you want to initiate the conversation with.
- Set the
App user ID
andApp thread ID
fields for the user. - Now you can create a new ticket as described above.
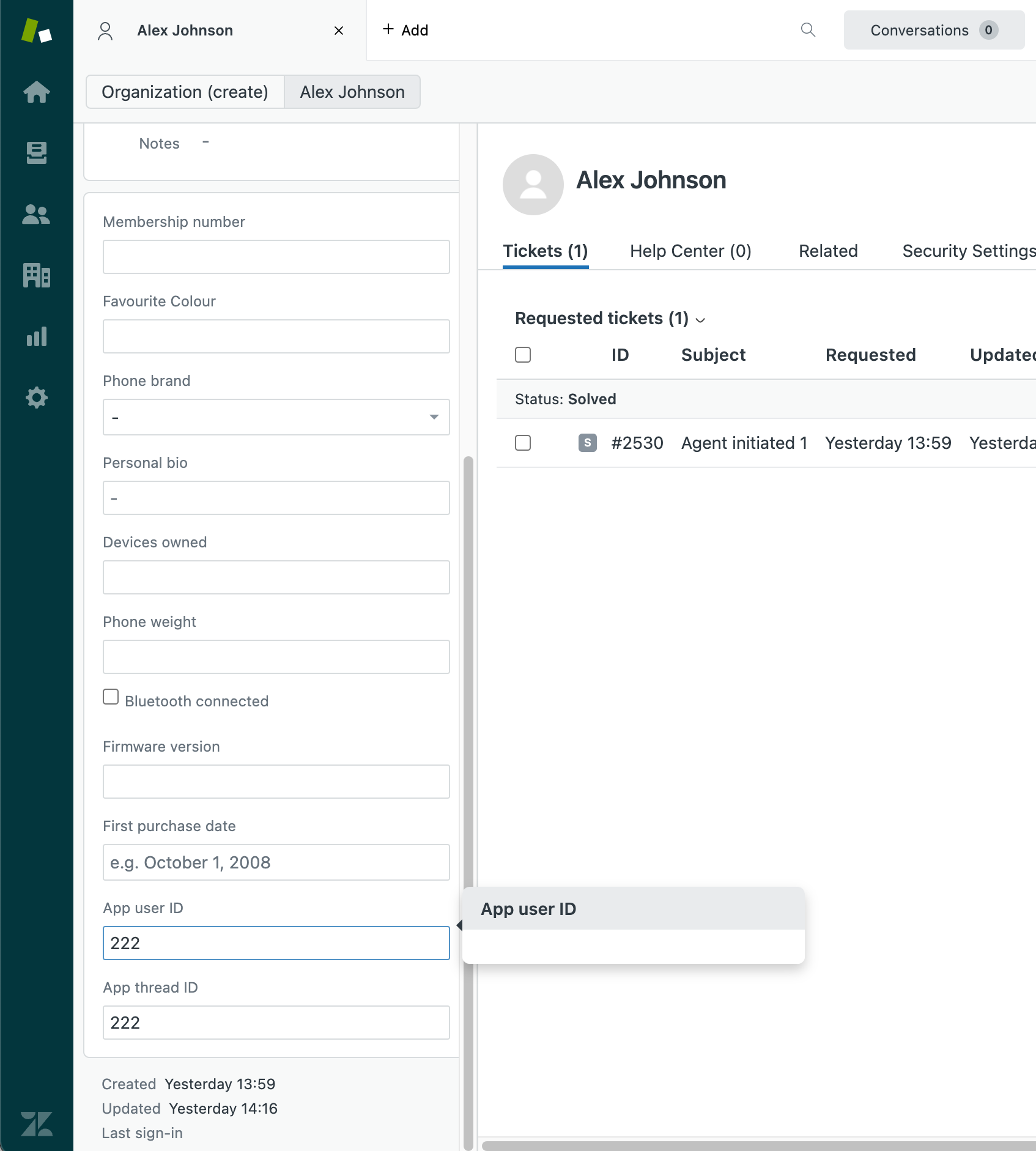
Updated 15 days ago