Component triggers
Implement complex behaviour by adding triggers to components
In the same way that flows can have triggers, built-in or custom, components can also have triggers.
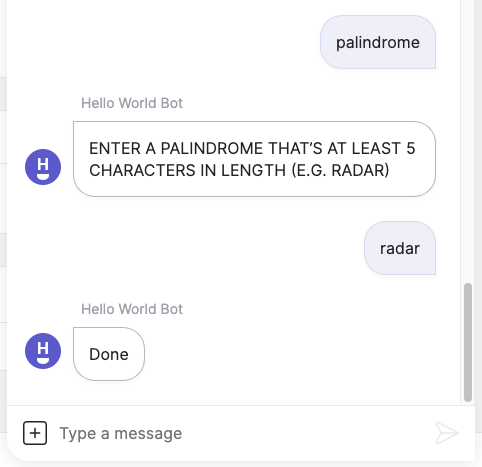
A custom component with a custom trigger that matches with palindromes (e.g. radar).
Use-cases
Component triggers can be used to:
- Improve non-UI integration experiences, like SMS and email.
- Add a context-sensitive Dialogflow trigger to an email form.
- Handle unprompted image input during ticket creation pre-chat instead of triggering a catchall flow or a flow with an image trigger.
Component triggers can only be triggered from within a flow when the user is at that step of the flow. In other words, component triggers cannot be triggered from outside of a flow.
Example 1: Built-in component with built-in trigger
Let's take a look at a basic example. By combining a say
component with a catchall
trigger, we can mimic the behaviour of an ask
component. Here's how.
1. Create the flow
In your app's flow
folder, create a new file called component_trigger_demo_1.yaml
and copy this code into it:
triggers:
- keyword: component_trigger_demo_1
steps:
- say: What's your name?
triggers:
- catchall
- say: Hi, (@ flow.result )!
Save the file, then upload it to the Grid by running these commands in your terminal:
meya format
meya push
2. Test it out
Example 2: Custom component with custom trigger
Here is a more interesting example using a custom component and custom trigger. Let's create a custom component that asks the user a question in uppercase letters. It will accept the user's input and continue the flow if they enter a palindrome (e.g. radar
), otherwise the flow will end.
1. Create the component
In your app's component
folder, create a folder called text
. Inside that folder create a file called say_upper.py
and copy this code into it:
from dataclasses import dataclass
from meya.component.element.interactive import InteractiveComponent
from meya.element.field import element_field
from meya.entry import Entry
from meya.text.event.say import SayEvent
from typing import List
from typing import Optional
@dataclass
class SayUpperComponent(InteractiveComponent):
say_upper: Optional[str] = element_field(signature=True)
async def start(self) -> List[Entry]:
say_event = SayEvent(text=self.say_upper.upper())
return self.respond(say_event)
2. Create the trigger
Now let's create the trigger.
In your app's root folder, create a folder called trigger
, if it doesn't already exist. Inside, create a folder called text. Within the
textfolder, create a file called
palindrome.py` and copy this code into it:
from dataclasses import dataclass
from meya.element.field import element_field
from meya.text.trigger import TextTrigger
from meya.trigger.element import TriggerMatchResult
@dataclass
class PalindromeTrigger(TextTrigger):
minimum: int = element_field(default=5)
async def match(self) -> TriggerMatchResult:
text = self.entry.text
if len(text) >= self.minimum and text == "".join(reversed(text)):
return self.succeed()
else:
return self.fail()
3. Create the flow
Finally, let's create a flow that uses the new trigger and component.
triggers:
- keyword: palindrome
steps:
- say_upper: Enter a palindrome that's at least 5 characters in length (e.g. radar)
triggers:
- type: trigger.text.palindrome
minimum: 5
- say: Done
Upload the new code by running these commands in your terminal:
meya format
meya push
4. Test it out
Navigate to your app's simulator and type palindrome
to launch the flow. Enter a valid palindrome to watch the flow continue. If you enter a non-palindrome, the flow will end instead.
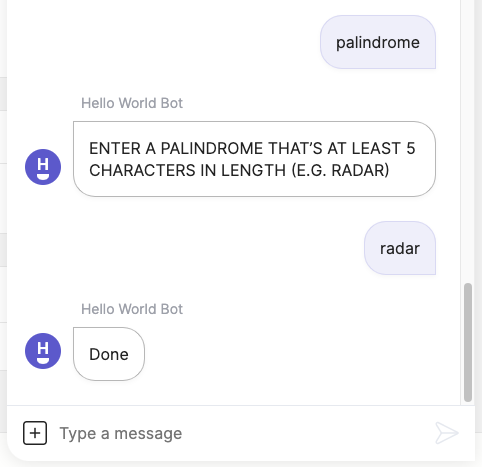
Consider what would have been involved with implementing this without the component trigger. We would have needed:
- an
ask
component to get the user's input,- a custom component to check if the input is a palindrome,
- another step to jump back to the
ask
component if the user did not enter a palindrome,- and a step label before the
ask
component so we can jump to it.Component triggers let you add interesting behaviour to your flows while remaining concise.
Updated almost 3 years ago