Git Workflow
How to manage your team's work effectively
Meya supports two typical development scenarios. The first relies on Meya's internal system to manage code, and the second which can be connected to external source control
Scenario 1
We refer to this as a "low code" workflow, as it's most appropriate when you are developing your code within the web console, or possibly from the CLI.
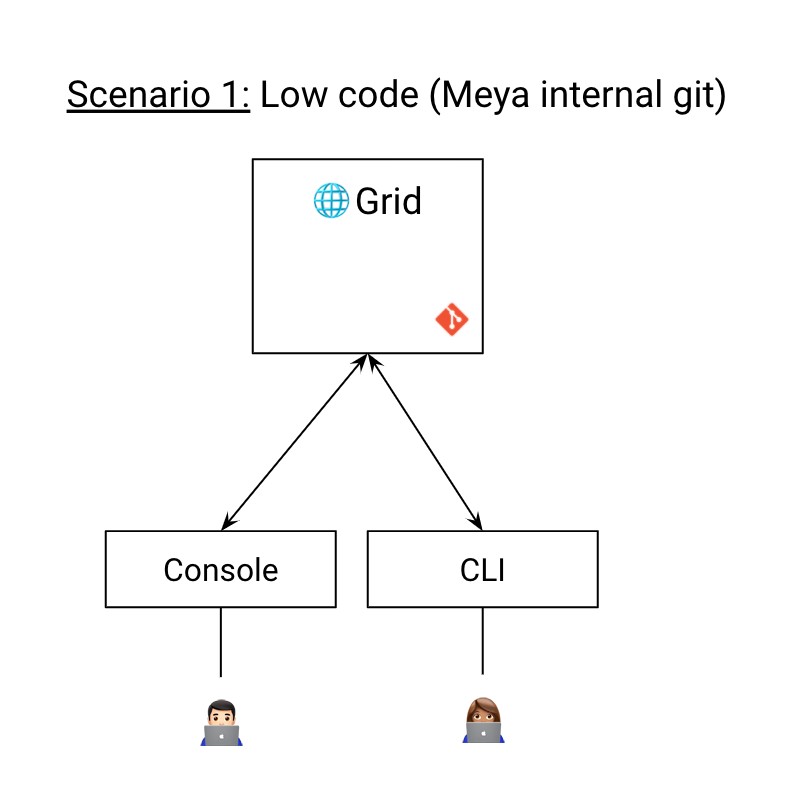
The low-code workflow.
In this workflow:
- The developer writes their code either in the web console or in their local environment.
- If they are working on their local environment, they'll use
meya push
to upload their changes to their app. - Testing is done either from the app's Simulator page, or from another integration.
- To move the code to a different app--so another developer can test, or to deploy the code to a production app--the developer runs
meya connect
to connect to another app, thenmeya push
to upload the code.
Re-connect to your development app!
If you deploy code to production using
meya connect
, don't forget to re-connect to your development app afterward. Otherwise, you could make unintended changes to your production app!
The main difference between this and scenario 2 is the lack of external source control.
Pros:
- Simple workflow.
- Great for solo developers.
- Great for low-code developers.
Cons:
- More difficult to share code between developers for review and testing.
- No automated testing or deployment.
- Harder to enforce proper coding.
- Harder to rollback bad commits.
- Using
meya connect
to share code can lead to changes being made to the wrong app if developers forget to re-connect to their dev app afterward.
Scenario 2
The "deep-code" approach is more advanced and is appropriate for teams of developers who want to apply good software-development practices to their app development using their preferred version control system.
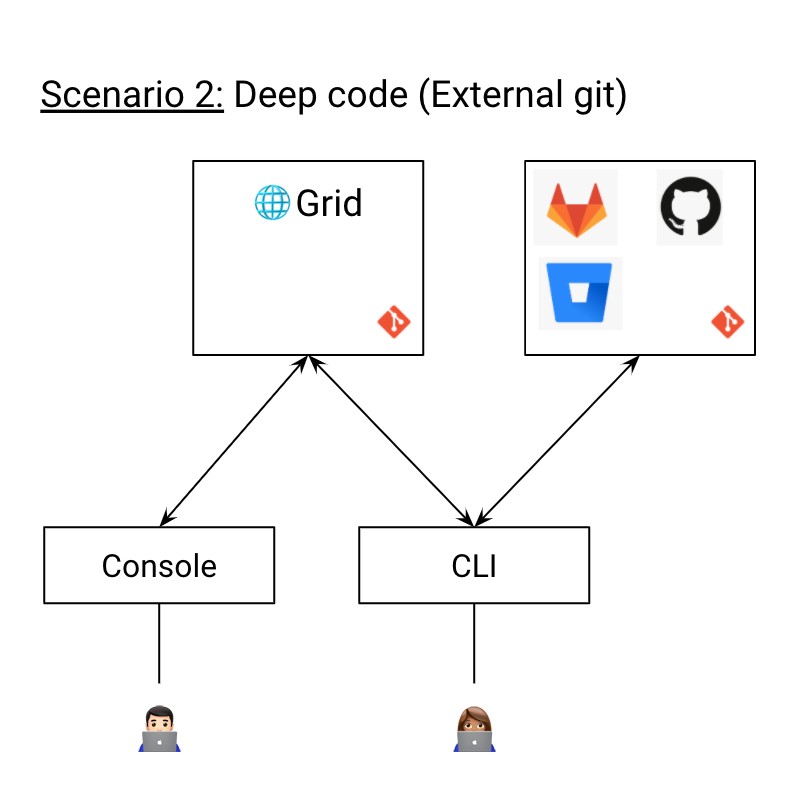
The deep-code workflow.
In this workflow:
- Each developer has their own Meya dev app which their local environment is connected to using
meya connect
. - When they make code changes, they use
meya push
to test them out in the Meya console. - When their code is working and they’re ready to commit it, they
git push
to a feature branch (i.e. not themain
/master
branch) - In Github, they open a PR for their work. You can use your normal PR process to make sure the code is reviewed.
- To test someone else’s work,
git pull
their branch to your local environment, then runmeya push
to push it to your own Meya dev app. - Once you approve the PR and merge it into
main
, the CD process will automatically deploy the new code to your production Meya app. See Deployment below for an example of how to implement CD using Github Actions.
Restrict access to the production app
In this approach, it is recommended that only the admin have edit access to the production Meya app. All other developers only need edit access to their own dev app (see image below). Modification of the production app should only happen through the CD process (see Deployment below).
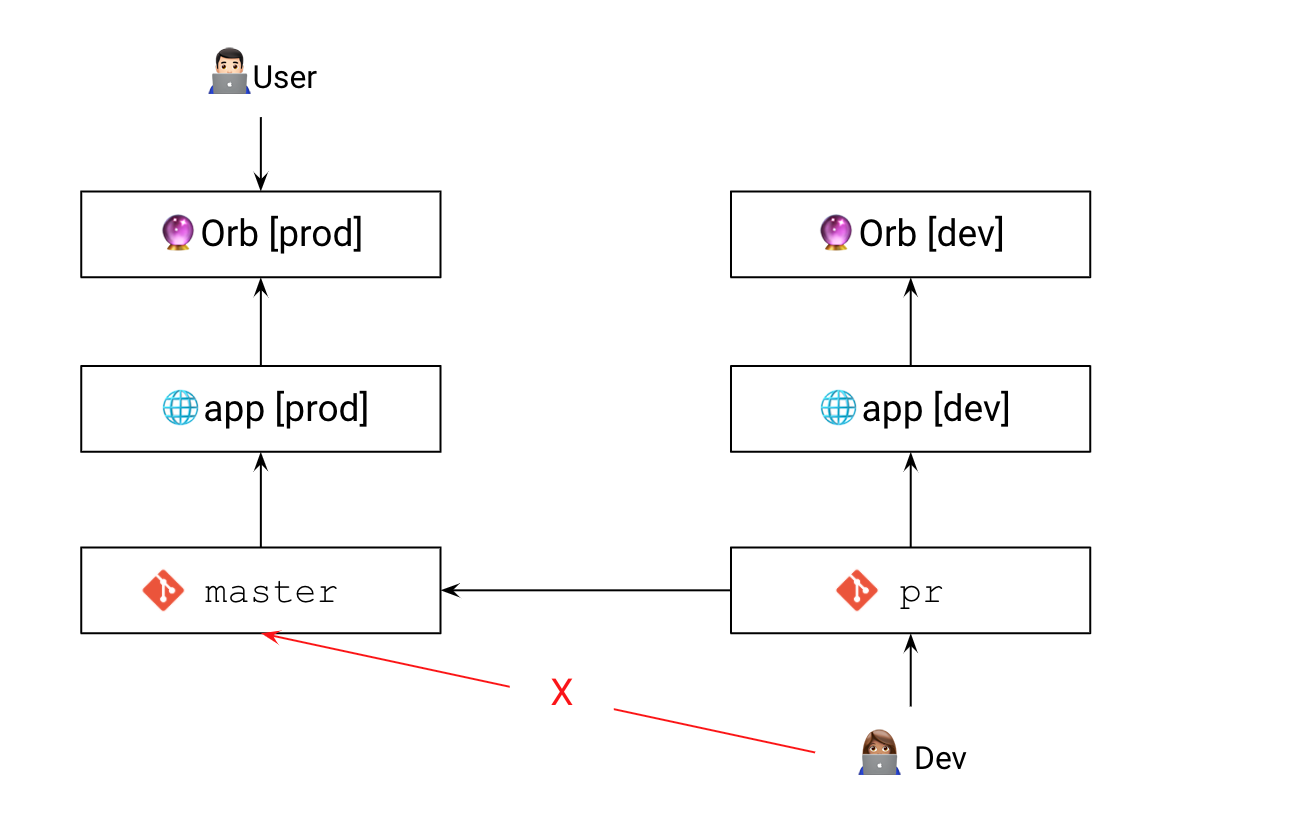
Developers should not modify the production app, or commit code directly to main
. Use feature branches and dev apps.
Pros:
- Great for teams of developers.
- Provides a way to back up your code off of the Meya platform.
- Can use your source control system to enforce proper coding using PR processes and restricting edit access of key branches.
- Makes it easy to see everyone's code even if you don't have access to the Meya platform.
- Makes testing someone's work straightforward: just
git pull
their feature branch, thenmeya push
to your dev app. - You can use version control to tag certain commits as releases
- Can easily rollback to a previous commit, if needed.
- You can setup a CI/CD pipeline to automatically test code and even push it to your production app once it's merged into the production branch.
- No confusion over what app you're connected to since all devs only work off of their own dev app,
git pull
ing other branches as needed. - The production app is protected since no developer has edit access.
Cons:
- More work to set up.
Deployment
In this section, you'll learn how you can use Github Actions to automatically test, build, and deploy your code.
Note that you are not restricted to using Github for your remote repos and CI/CD process. All examples on this site, though, do use Github.
Add repo secrets
In your Github repo, navigate to the Settings tab. Choose Secrets from the left-hand menu, then click Add repository secret.
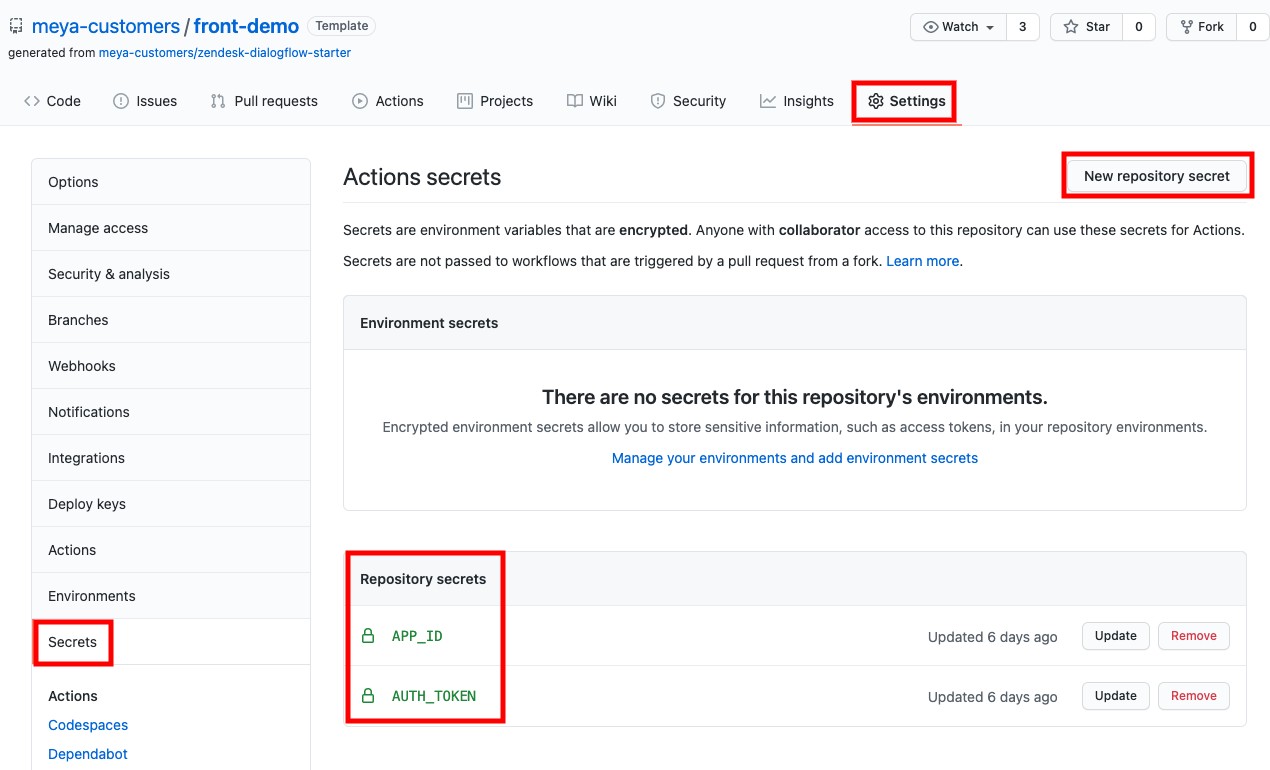
Create two secrets:
APP_ID
: The value should be your production Meya app, not a dev app.AUTH_TOKEN
: Your Meya auth token.
Permissions
Note that your token needs to have permission to modify the production app and vault. To check this:
- Navigating to your Team Members page.
- Check what Team you're a part of.
- Open the Team's page and verify it has the appropriate access to the production app in the App permissions section.
Add the workflow
In your Github repo, select the Actions tab, then click the set up a workflow yourself link.
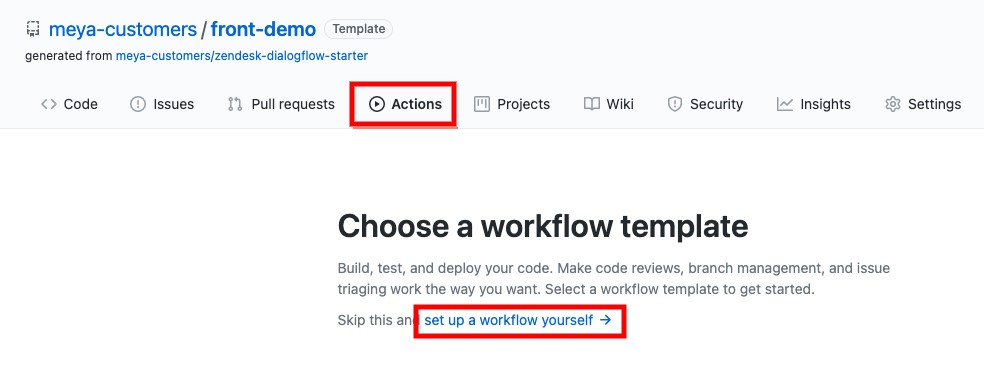
Give the workflow a name, then replace the existing code with this code:
# This workflow will install Python and Meya dependencies, runs tests and code formatting check
name: Meya build
on:
repository_dispatch:
push:
branches:
- '**'
jobs:
build-and-deploy:
runs-on: ubuntu-latest
steps:
- uses: actions/checkout@v2
- name: Set up Python 3.8
uses: actions/setup-python@v1
with:
python-version: 3.8
- name: Install dependencies
run: |
python -m pip install --upgrade pip
pip install --upgrade \
--extra-index-url https://meya:${{ secrets.auth_token }}@grid.meya.ai/registry/pypi \
"pygit2==1.1.1" \
"meya-sdk>=2.0.0" \
"meya-cli>=2.0.0"
- name: Authenticate using Meya auth token
run: |
meya auth add --grid-url https://grid.meya.ai --auth-token ${{ secrets.auth_token }}
- name: Connect to production app
run: |
meya connect --grid-url https://grid.meya.ai --app-id ${{ secrets.app_id }}
- name: Check code syntax and formatting
run: meya check
- name: Run tests
run: meya test
- name: Deploy
if: github.ref == 'refs/heads/main'
run: meya push --force --build-image
Line 8: This workflow will run when code is pushed to any branch.
Lines 12-26: Some initial setup is done, including installing Python 3.8 and the required dependencies using pip
.
Lines 27-32: The workflow uses your auth token and app ID to authenticate with Meya and connect to your production app.
Lines 33-36: Checks and tests are run to verify that the code is properly formatted and works.
It's always a good idea to run
meya format
,meya check
, andmeya test
before committing code, but in case you or your teammates forget, this will make sure errors are highlighted in Github.
Lines 37-39: The workflow will now push the code to your production app only if code was pushed to the main
branch.
main
is the preferred term for your main production branch. It was formerly called themaster
branch. If you still use the termmaster
, be sure to update line 38 torefs/heads/master
.
Save the workflow.
Updated 25 days ago