Page context
Pass page context from Orb to BFML
Passing contextual information to your app allows you to make your app aware of the page it’s on and the user it’s interacting with. There are many benefits to providing contextual information:
- Provide your app with information about the user so you don’t have to ask them for it again.
- Let your sales rep know what product the user was looking at when they asked to talk to sales.
- Use the user’s location to connect them to a local sales rep or the support team closest to their time zone or geolocation.
- Provide your app with information about how the user is using your web platform:
-
- Your free trial is about to expire. Do you want to add a plan?
-
- You haven’t tried feature X yet. Would you like to view a tutorial?
-
- I see you got error message X. Here are some troubleshooting tips.
- Have your bot respond to the user in their own language (see navigator.language).
In this guide, you’ll learn how to pass this kind of data to your app using the Orb integration.
These examples assume you have an Orb integration set up for your app.
Example 1
In this example, we’ll pass in a hardcoded value to the bot using the pageContext
property.
- Create a new Codepen and copy this code into the HTML box:
<script type="text/javascript">
window.orbConfig = {
connectionOptions: {
gridUrl: "https://grid.meya.ai",
appId: "APP_ID",
integrationId: "integration.orb",
pageContext: {
count: 9
}
},
windowApi: true,
};
(function(){
var script = document.createElement("script");
script.type = "text/javascript";
script.async = true;
script.src = "https://cdn.meya.ai/v2/orb.js";
document.body.appendChild(script);
var fontStyleSheet = document.createElement("link");
fontStyleSheet.rel = "stylesheet";
fontStyleSheet.href = "https://cdn.meya.ai/font/inter.css";
document.body.appendChild(fontStyleSheet);
})();
</script>
Make sure to update the
APP_ID
value to your app’s ID.
Any data you pass to the Orb in the
pageContext
property will appear inflow
scope atflow.context
.
Save your changes.
- In your Meya app, create a new flow called
context.yaml
and copy this code into it:
triggers:
- type: page_open
steps:
- say: Context => (@ flow.context )
Since
flow
scope data does not persist, you may want to use auser_set
orthread_set
component to save this data for later use.Further reading: How to Store Scope Data
Save the file and push it to the Grid:
meya format
meya push
- Refresh the browser tab where Codepen is running. The Orb should open, run our new
context.yaml
flow, and display the context information we passed in.
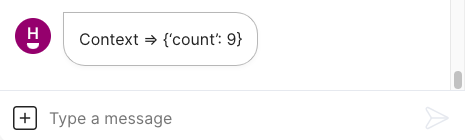
Example 2
In this example, you’ll create a mock e-commerce web page with a sample product. Then you’ll add the Orb and pass in product data scraped from the page so the app knows what product the user is looking at.
- Create a new Codepen and copy this code into the HTML box:
<head>
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="https://cdn.jsdelivr.net/npm/[email protected]/css/bulma.min.css">
</head>
<body>
<section class="section">
<div id="product" class="container">
<h1 id="product-name" class="title is-size-3 has-text-centered">Widget Pro</h1>
<p id="product-price" class="subtitle has-text-centered">$<span id="product-price-value">9.99</span></p>
<div class="columns mt-6">
<div id="product-image" class="column">
<img class="image" style="margin-left: auto; margin-right: auto; max-width: 300px;" src="https://www.windowcleaningworld.com/assets/full/GDWIDGET.jpg?20200709030844">
</div>
<div id="product-description" class="column">
<p class="block">
Lorem ipsum dolor sit amet, consectetur adipiscing elit. Ut semper finibus consequat. Vivamus venenatis fringilla magna. Praesent vel pulvinar leo, et porta odio. Quisque ut augue vel quam pulvinar gravida. Integer nec erat eget purus tempor facilisis sit amet a nisi. Morbi sed auctor lectus. Nam laoreet placerat consequat. Cras vitae nulla velit. Nunc gravida, massa et bibendum aliquet, turpis elit bibendum mi, in lacinia dui tortor vitae odio. Suspendisse finibus metus nec sem tempor convallis. Fusce vulputate mi mi, et vehicula lectus feugiat id.
</p>
<p class="block">
Nulla facilisi. Curabitur est elit, euismod eget lectus ac, iaculis consectetur dui. Mauris scelerisque faucibus auctor. Nam consectetur non felis at fringilla.
</p>
<button class="button is-primary">Buy for $9.99</button>
</div>
</div>
</section>
</body>
The web page should look something like this:
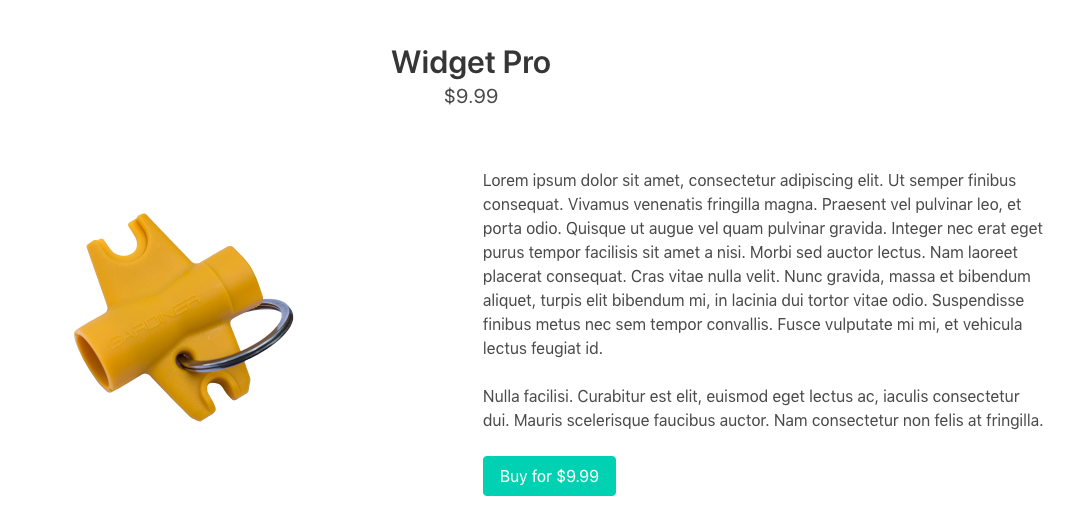
- Add in the Orb JS snippet immediately before the closing
</body>
tag:
<script type="text/javascript">
window.orbConfig = {
connectionOptions: {
gridUrl: "https://grid.meya.ai",
appId: "APP_ID",
integrationId: "integration.orb",
},
windowApi: true,
};
(function(){
var script = document.createElement("script");
script.type = "text/javascript";
script.async = true;
script.src = "https://cdn.meya.ai/v2/orb.js";
document.body.appendChild(script);
var fontStyleSheet = document.createElement("link");
fontStyleSheet.rel = "stylesheet";
fontStyleSheet.href = "https://cdn.meya.ai/font/inter.css";
document.body.appendChild(fontStyleSheet);
})();
</script>
Make sure to update the
APP_ID
value to your app’s ID.
- Now, let’s add some code to our JS snippet that reads product information from the page.
Place this code immediately after the <script type=”text/javascript”>
tag:
var product = {};
product.name = document.getElementById("product-name").innerHTML;
product.price = parseFloat(document.getElementById("product-price-value").innerHTML);
- In the
connectionOptions
object, add this as a new property:
pageContext: {
product: product
}
The finished JS snippet should look like this:
<script type="text/javascript">
var product = {};
product.name = document.getElementById("product-name").innerHTML;
product.price = parseFloat(document.getElementById("product-price-value").innerHTML);
window.orbConfig = {
connectionOptions: {
gridUrl: "https://grid.meya.ai",
appId: "APP_ID",
integrationId: "integration.orb",
pageContext: {
product: product
}
},
windowApi: true,
};
(function(){
var script = document.createElement("script");
script.type = "text/javascript";
script.async = true;
script.src = "https://cdn.meya.ai/v2/orb.js";
document.body.appendChild(script);
var fontStyleSheet = document.createElement("link");
fontStyleSheet.rel = "stylesheet";
fontStyleSheet.href = "https://cdn.meya.ai/font/inter.css";
document.body.appendChild(fontStyleSheet);
})();
</script>
Save the Codepen.
- Finally, let’s update the
context.yaml
flow:
triggers:
- type: page_open
steps:
- say: Would you like me to check if (@ flow.context.product.name ) is available at a store near you?
Push the updated code to the Grid:
meya format
meya push
Refresh your Codepen to test it out:
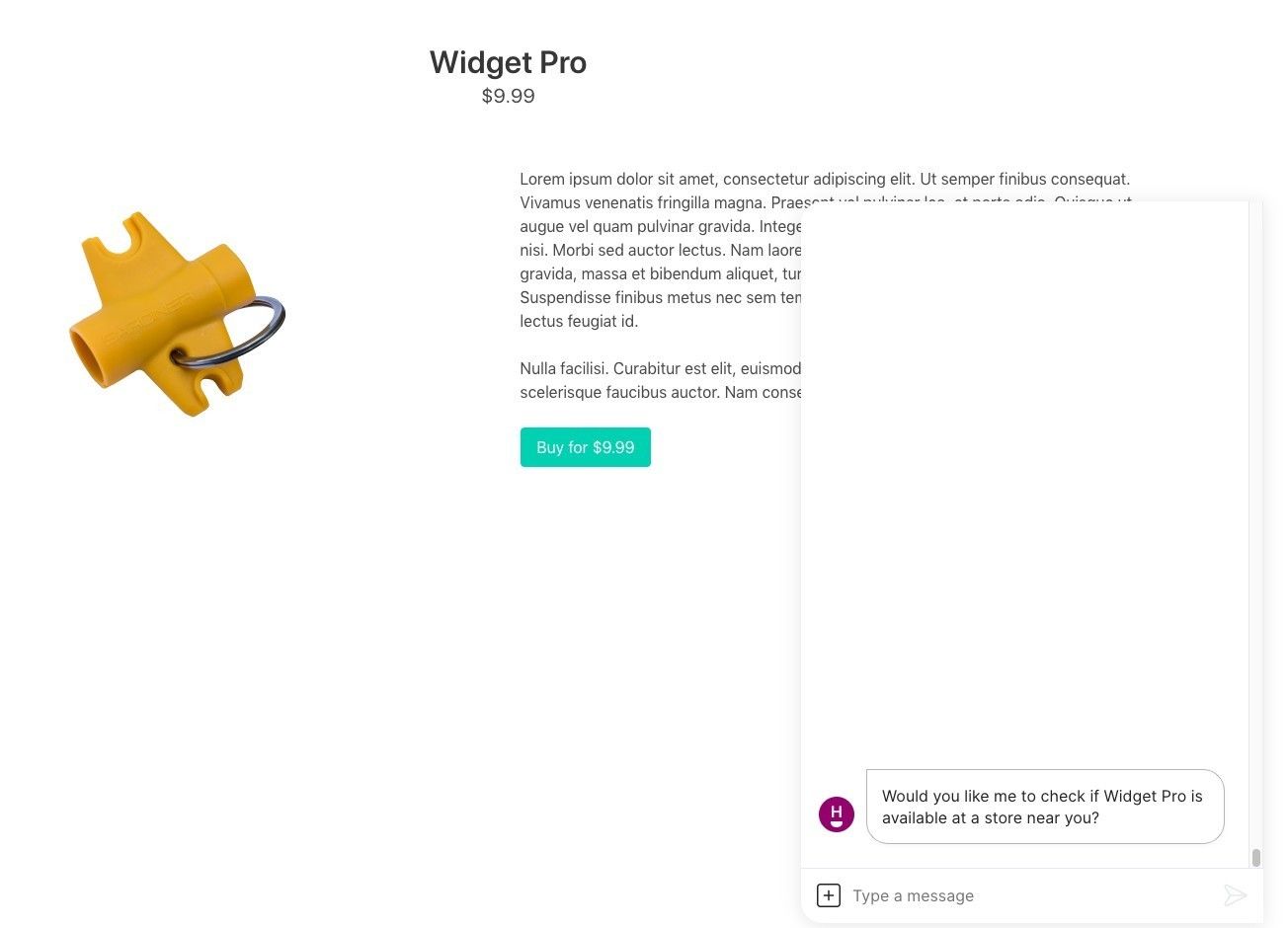
Updated 15 days ago