Python Dependencies
Create a requirements.txt file to include your own Python dependencies
With the Python dependencies feature, you can write your own requirements.txt file specifying the dependencies you wish to include in your bot’s environment.
Use-cases
- You want to add a popular Python package to your bot.
- You want to add a Python dependency you wrote for use in a custom component.
- Code re-use: You want to add a Github-hosted Python dependency.
- Cloning a bot: When you clone a bot, sync from GitHub, or import a bot .zip file, requirements.txt will be included.
Built-in dependencies
There are eight dependencies that come pre-installed for your bot to use. All you have to do is import them in your custom component.
boto3
requests
arrow
geopy
bs4
twilio
google-api-python-client
jwt
With the Python dependencies feature, you can increase your bot's capabilities.
Importing and exporting requirements.txt
- requirements.txt is exported when you download a .zip of your bot and is imported when loading a .zip into Meya.
- requirements.txt is committed to GitHub along with the rest of your bot’s code, and imported when syncing from GitHub.
- requirements.txt is copied when cloning a bot.
Bot redeployment
Anytime requirements.txt is imported, created, or edited, your bot will re-deploy. Depending on the number and size of the dependencies, as well as your bot’s code, this may take several minutes.
A quick example
Let's take a look at how Python dependencies can come in handy. In this example we'll perform some matrix math using the Python library, NumPy
In a new or existing bot, create a new flow and enter the following flow code.
states:
start:
component: matrix_math
When you save, you’ll be reminded to create the component your flow refers to. Create the matrix_math
component, enter the following code, and click Save.
from meya import Component
import numpy as np
class MatrixMath(Component):
def start(self):
a = np.arange(15).reshape(3, 5)
print a
message = self.create_message(text="Testing Python dependencies")
return self.respond(message=message, action="next")
NumPy is not one of the Python modules installed in your bot’s environment by default, so when you click Unit Test you’ll get an error.
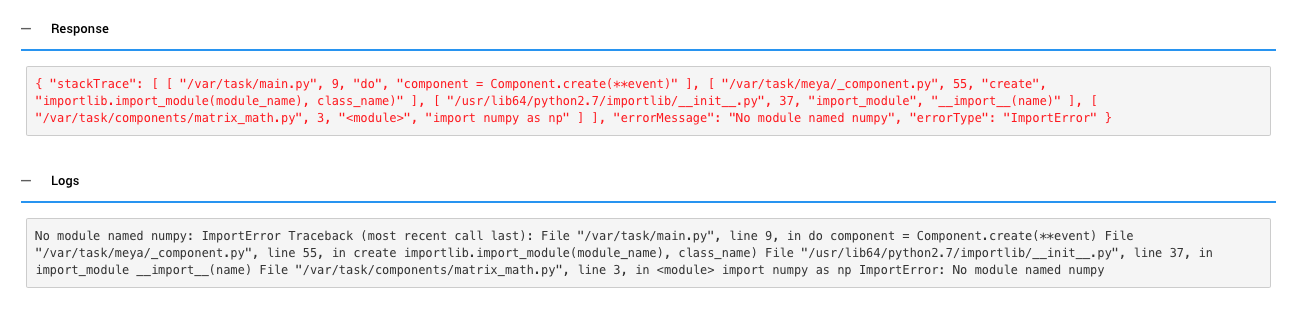
An error message during unit testing.
Let’s create a requirements.txt file to tell the platform what Python dependencies need to be installed. Behind the scenes, the platform will pip install these modules.
Creating requirements.txt
Within Bot Studio, look for the new Config section of the file explorer to the left of the code editor. Click it and select Add custom dependencies.
Note
The Custom Python Dependencies feature is only available to Enterprise customers.
Enter the word numpy
in the file, and click Save. A message will appear alerting you that your bot is re-deploying, and that it can take 30-120 seconds to complete.
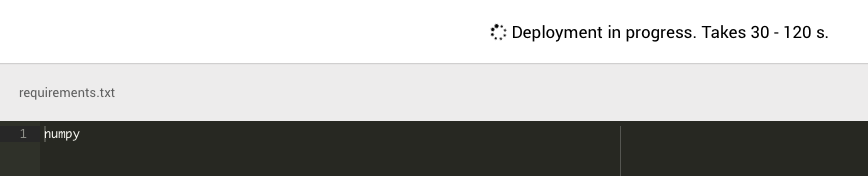
Dependency deployment is in progress.
Bot redeployment
Every time you save the requirements.txt file, your bot will re-deploy. Depending on the number of dependencies that need to be installed, and your bot’s code, this can take some time. If you save your requirements.txt file and immediately go back and try to run your custom components, they may still fail. Don’t worry! All you have to do is wait a little longer.
If you see the following message, or no message at all, within the requirements.txt editor, your bot has successfully re-deployed!

Dependency deployment was successful.
Go back to the matrix_math.py component and click Unit Test. The test should pass, and in the Logs section you’ll see the matrix printed.
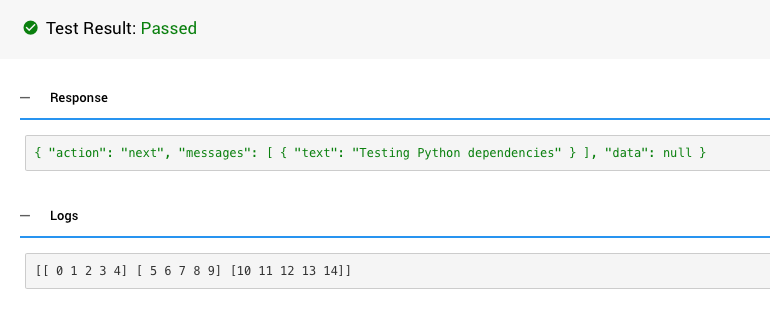
The dependency now works.
Installing a Python dependency from a GitHub repo
To install a public GitHub-hosted dependency, use the following syntax in your requirements.txt file:
git+https://github.com/meya-ai/pip-bot-example
A private Github-hosted dependency requires the following syntax, after acquiring a personal access token from Settings -> Developer settings -> Personal Access Token on Github:
git+https://<paste access token here>@github.com/<repo owner>/<private repo>
If you want to test the above dependency, create a custom component and enter the copy-paste the following code and click Save.
from meya import Component
from utilities import foo, multiply
class GitHubPip(Component):
def start(self):
print foo()
print multiply(10, 20)
return self.respond()
The pip-bot-example repo contains a utilities.py module containing two functions: multiply() and foo().
Click Unit Test. The test should pass, and you should see the text bar 200
in the Logs section.
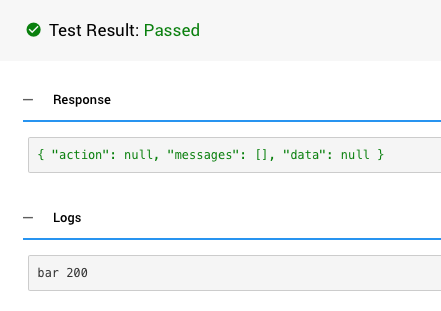
The component passes unit testing.
Creating a Python dependency
You may want to share your bot's functions with other bots. While you could recreate a custom component that holds the functions you want to share, and then copy-paste that into your new bots, the Python Dependencies feature offers a more elegant solution. By creating your own Python module and hosting it on a GitHub repo, you can easily share your bot's functions. Let's see how.
You can use this simple dependency as a template for your own: pip-bot-example
Create a new folder. Rename the folder to match your module's name. Move your module into the folder. Rename the Python file to __init__.py
(note the double underscores on each side).
Move into the parent directory and create a file called setup.py
. Open the file in a text editor and copy-paste the following code.
from setuptools import setup
VERSION = '1.0.0'
description = (
'BASIC_DESCRIPTION_OF_THIS_PACKAGE'
)
install_requires = []
packages = [
'YOUR_MODULE_NAME' # Replace with the name of your Python module.
]
setup(
name='YOUR_DEPENDENCY_NAME',
version=VERSION,
description=description,
url='YOUR_GITHUB_REPO_URL',
author='YOUR_NAME',
author_email='YOUR_EMAIL',
license='None',
packages=packages,
install_requires=install_requires,
zip_safe=False)
Make sure you update the capitalized strings in setup.py to match your module's actual information.
If you're stuck...
If you get stuck and aren't sure what information goes where, refer back to the pip-bot-example setup.py file.
Create a new GitHub repo without any files in it (e.g. README.md, .gitignore). On your local machine, initialize a git repo and push the code to your GitHub repo.
New to git?
If you're unfamiliar with git or GitHub, check out these instructions.
Congratulations!
You should now be able to add your Python dependency to your bot's requirements.txt file in Meya. Use the instructions in the Installing a Python dependency from a GitHub repo section above to see how to use your dependency in a custom component.
Updated almost 7 years ago