Flow Details
Step-by-step execution.
A flow is a set of states that will execute in a defined order, executing components or other nested flows at each step. In the Meya Bot Platform, you will define a flow using YAML syntax.
# * denotes default value
triggers:
- type: {type}
properties:
{property1}: {value1}
{property2}: {value2}
route: {true|false*} # use when intents match state names
intents:
start: {state1}
{alt_intent1}: {state2}
{alt_intent2}: {state3}
...
states:
{state1}:
component: {component_name}
properties:
{key1}: {value1}
{key2}: {value2}
...
transitions:
{action1}: {state1}
{action2}: {state2}
...
{state2}:
flow: {flow_name}
intent: {intent_name}
data:
{key1}: {value1}
{key2}: {value2}
...
properties:
{key1}: {value1}
{key2}: {value2}
...
transitions:
{action1}: {state1}
{action2}: {state2}
...
return: {true|false*}
States can have component OR flow
Each state can invoke a
component
or aflow
, but not both. This will cause errors.
Fields
intents: A map of custom intents. The key is the name of the intent, and the value should match a state name in the flow. start
is the default intent, and if no intents are specified the first state will be invoked. optional
component: the name of the component to execute. Either flow or component is required. required
flow: the name of the flow to execute (nested flows). Either flow or component is required.
properties: collection of key / value pairs to pass to the component or flow. optional
transitions: Flow and components output actions (ie. "next" or "stop" or "success") that can be used for flow control. If omitted, the next step in the flow will execute automatically optional
return: Boolean set to true
(false
is ignored). Explicitly signifies the the end of the flow. Can be used when the last logical state doesn't occur at the end of the yaml file. optional
Example
The following example:
- Asks the user their age
- If they are younger than 18, the bot responds "Sorry"
- If they are older than 18, the bot responds "Congratulations"
states:
ask:
component: meya.input_integer
properties:
text: How old are you?
output: age
check:
component: age_filter
transitions:
under: block
over: allow
block:
component: meya.text
properties:
text: Sorry, you have to be 18 to continue.
return: true
allow:
component: meya.text
properties:
text: Congratulations! You can continue.
return: true
Note: age_filter is a custom component that checks the age
variable. It must be implemented in order for the check_age
flow to work.
from meya import Component
class AgeFilter(Component):
def start(self):
# read in the age, and default to `0` if invalid or missing
age = self.db.flow.get('age')
try:
age = int(age)
except:
age = 0
# check the age
if age >= 18:
action = "over"
else:
action = "under"
# the action determines which transition is invoked
# note that no message is returned, just an action
return self.respond(message=None, action=action)
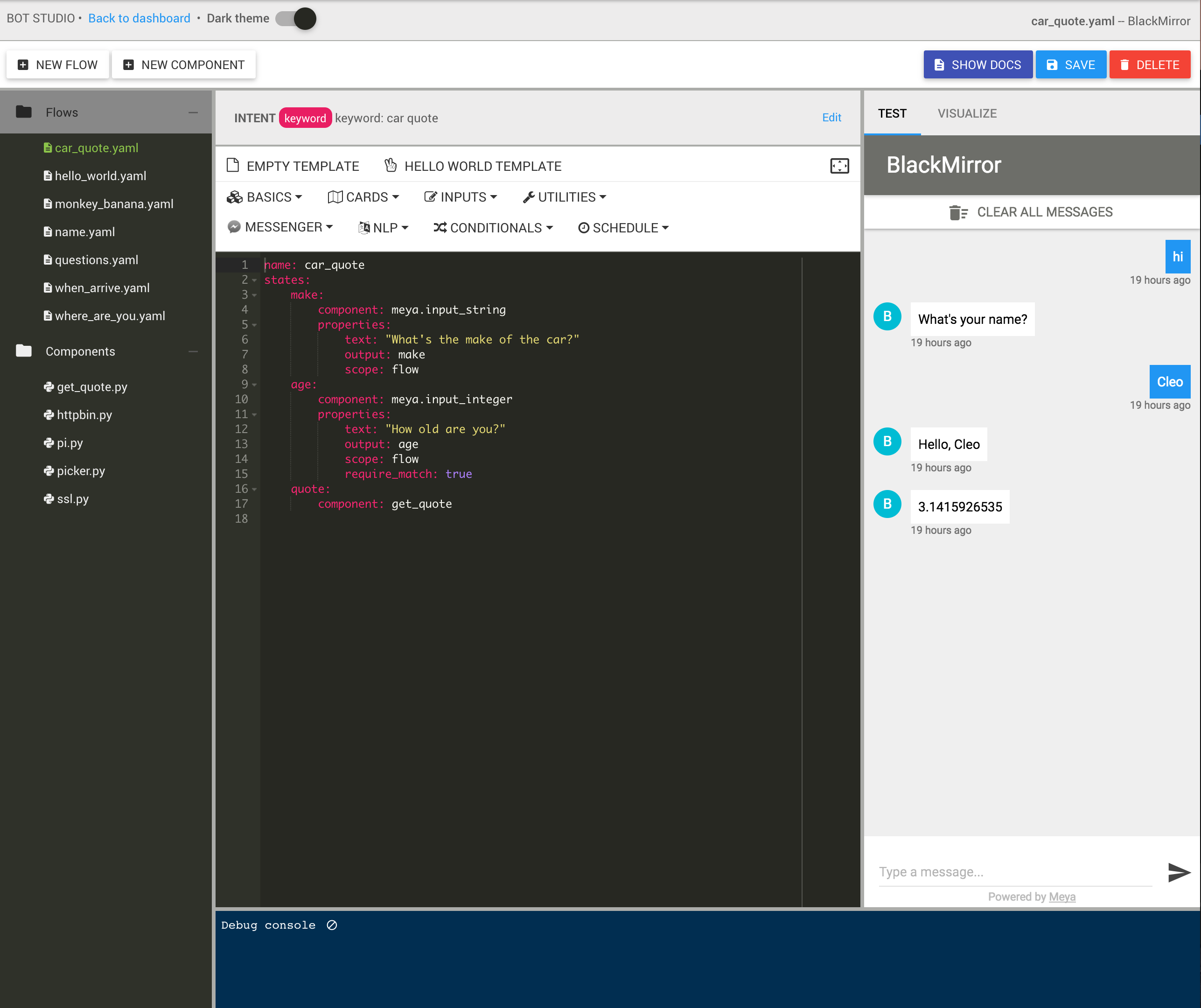
Editing a flow in the web IDE
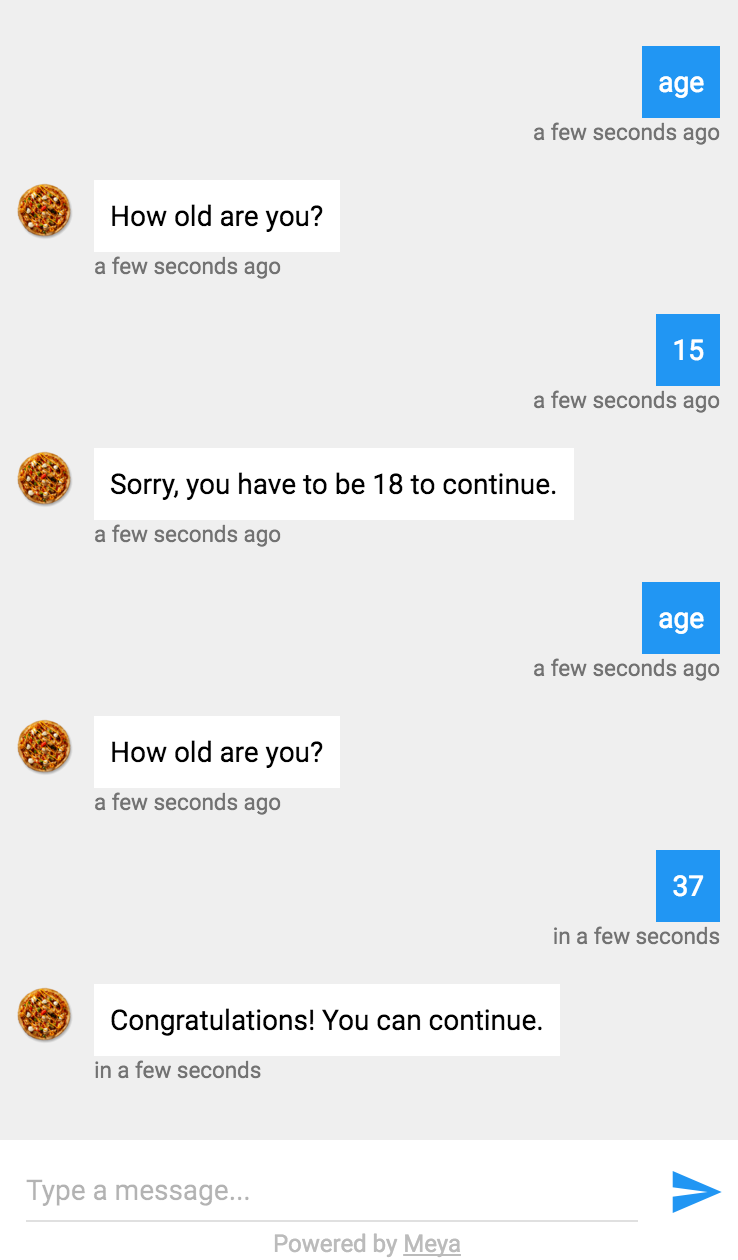
The age checker example.
Maximum number of states per run
A maximum of 25 states can execute in a given "run" of a flow. For example, this could be 25 different states executing once each, or the same state repeating 25 times. This is to prevent infinite loops from occurring.
If you notice some states in your flow aren't executing, verify that you aren't running into this limit.
Updated over 5 years ago