Bot Building Blocks
Learn the basic concepts and definitions used in bot-building.
Before you dive in to the actual code, it's important that you understand the basic terminology used on the Meya platform, and the components you can combine to define very complex and intelligent behaviour in your bot.
User: Someone who is talking to your bot.
Integration: An integration is a connection between your bot and another platform, such as Facebook Messenger or Intercom, which let's users interact with your bot on that platform.
Triggers
When a user talks to your bot, it needs to understand what they meant to say, regardless of whether they spelled it correctly or used the most logical phrasing. For example, a user who says "What's up?" is probably greeting your bot, not asking what is literally "up". In other words, your bot must understand the user's intent. Triggers allow you to specify which intents should trigger the flow. Here are a few examples of trigger types your bot might make use of:
- An NLU trigger that let's your bot use Natural Language Understanding to intelligently detect a user's intent from a broader variety of input.
- A "catch-all" trigger that let's you handle user input that doesn't match any other intent. This allows your bot to gracefully handle a user's unknown intent with a friendly message instead of simply not responding.
- A keyword trigger that let's your developers access hidden flows for testing and debugging purposes by typing an unlikely sequence of characters, like
_t3st!ng
Triggers are core concepts in bot-building. They are used to determine which sequence of actions your bot should perform. You can find a full explanation of the trigger types available here.
Flows
On the Meya platform, we refer to a sequence of related actions as a flow. Each action is called a state. Each flow will have one or more states, or actions, for the bot to perform. Here are a few example flows your bot might have:
- A "welcome" flow that says "Hi" to the user, asks them their name, and stores it in a database your bot can access from other flows.
- A "create a reservation" flow that asks the user for the time they want to reserve a table at your restaurant, how many people they're expecting, and their phone number, and makes an API call to your reservation platform to save the data.
- A "transfer to agent" flow that allows the user to talk to a human if necessary.
Flows contain related actions
Each flow should have its own purpose. This makes debugging your flows easier, as well as moving from one flow to another. There are no limits on the number of flows you can have.
Components
A state can either use a component, or start another flow. A component describes the action your bot will perform. In Meya, there are components to:
- Display text, images, or videos to the user;
- Ask the user to enter some text, mark their location, or upload an image;
- Set and read information about the user in your bot's database;
- Launch other flows from within the current flow;
- Schedule a flow to occur at a later point in time.
There are many more actions your bot can perform using the built-in set of components. And if those aren't enough, you can develop your own custom components in Python!
Transitions
The most basic flows follow a linear path, like this one.
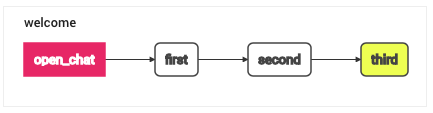
A basic flow.
This flow has a trigger (open_chat
) that launches the flow. It begins with the first state, transitions to the second, and finally, the third. The actual flow code looks like this:
states:
first:
component: meya.text
properties:
text: Hi!
second:
component: meya.text
properties:
text: I am a...
third:
component: meya.text
properties:
text: ...bot!
By default, your bot will perform the next action in your flow until it reaches the end of the flow. More complex sequences are possible using transitions. Transitions tell your bot what action within the flow to execute next based on the value of a variable, a user's input, a button click, and more.
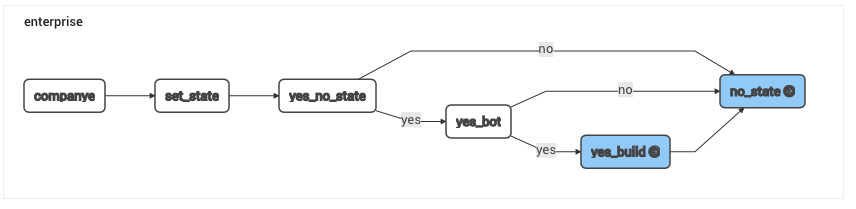
A more complex flow.
Here's what the flow code looks like:
states:
company:
component: meya.text
properties:
text: Hi, I am a bot!
set_state:
component: meya.set
properties:
key: new_user
value: true
scope: flow
yes_no_state:
component: meya.nlp_yes_no
properties:
text: Have you used a bot before?
transitions:
'yes': yes_bot
'no': no_state
yes_bot:
component: meya.nlp_yes_no
properties:
text: Have you ever built a bot?
transitions:
'yes': yes_build
'no': no_state
yes_build:
component: meya.text
properties:
text: Awesome!
no_state:
component: meya.text
properties:
text: Well, get ready!
return: true
Notice the transitions section in the yes_no_state. This tells the bot that if the user types yes
, go to the yes_bot state next; if they type no
, go to the no_state.
If you haven't already, be sure to check out this video tutorial, "Your First Bot":
Updated almost 7 years ago
Now that you know some basic definitions and core concepts, let's get familiar with the user interface you'll be using to build your bots.