NLP components
Quick example
states:
welcome:
component: meya.text
properties:
text: "Let's test Dialogflow."
dialogflow_question:
component: meya.dialogflow
properties:
text: "Try saying 'Do you provide financing?' or 'Do you accept insurance?'"
project_id: XXXX
language: en
error_message: "I don't understand 🤔. Try again."
min_confidence: 0.8
require_match: true
transitions:
financing: financing_state
insurance: insurance_state
financing_state:
component: meya.text
properties:
text: "Yes, we do provide financing."
return: true
insurance_state:
component: meya.text
properties:
text: "Yes, we do accept insurance."
return: true
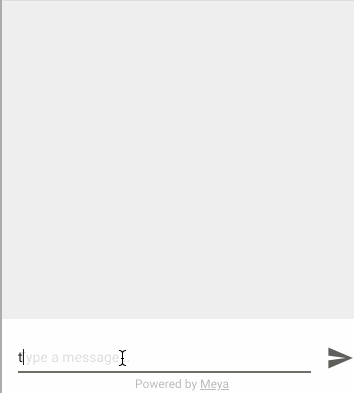
Working example of Dialogflow transition.
Intents are used to transition
In the
transitions
section of the code snippet above, bothfinancing
andinsurance
must be intents you've created in your NLU agent. If they don't exist, this code snippet will not work.Similarly, any NLU intent you want to handle in this component must have an entry in the
transitions
section, otherwise nothing will happen when the user expresses that intent.
Flow scope variables returned by the NLU agent
All of the NLU agents (Dialogflow, api.ai, luis.ai, and wit.ai) set several variables on the flow
scope related to the NLU intent matching process. These variables can be accessed from within a flow using Mustache syntax: {{ flow._intent }}
, or from a Python component using self.db.flow.get("_intent")
.
Variable | Description |
---|---|
_confidence | The agent's confidence in the match. |
_intent | The intent the Dialogflow agent matched. |
value | The user's original text. |
fulfillment.speech | The agent's response, if you've provided the app with responses. |
parameters.simplified | The query submitted to the agent. |
source | The source agent. |
meya.input_cms
Accepts input from the user and matches it against a Bot CMS-trained NLU agent. It also extracts entity data and makes that available on the flow
scope.
Property | Description | |
---|---|---|
text | The text to output to the user. | |
speech | Text to speak to the user. This field also accepts SSML markup to customize pronunciation. | Optional |
space | The space, or folder, in which the key is stored in Bot CMS. | Required |
key | The key, or intent, which will be returned as an action. | Required |
language | The two character language identifier. Example: en | Optional |
min_confidence | The minimum confidence to match the entity. | Optional. Default: 0.67 |
detect_language | If true , {{ flow.language }} will be set to the language the NLU agent detected from the user's input. | Optional. Default: false |
require_match | If false the flow will return an action no_match that you can use to transition to another state. | Optional. Default: true |
scope | Where to store the intent and entity data. One of flow , user , or bot . | Optional. Default: flow |
buttons | An array of Buttons | Optional |
error_message | Only displayed if require_match is true and the user's input does not match an intent. | Optional |
states:
input_cms_state:
component: meya.input_cms
properties:
text: "What would you like to update?"
space: "change"
key: "phone"
language: "en"
error_message: "I don't understand"
require_match: false
min_confidence: 0.8
detect_language: true
buttons:
- text: "My phone number"
action: phone
- text: "Something else"
action: no_match
transitions:
phone: update_phone_number
no_match: everything_else
update_phone_number:
component: meya.text
properties:
text: "Okay, let's update your phone number"
return: true
everything_else:
component: meya.text
properties:
text: "You said something else"
return: true
Transitions
next: the default transition for an answer (if not present, flow will transition to the next state in sequence)
no_match: the state to transition to if require_match
is false
and the user inputs something that doesn't match an intent, or matches an intent other than the one specified in this component.
The captured user intent will be available as
flow.intent
later in the next step(s) of your flow.
meya.dialogflow
Accepts input from the user and matches it against a pre-trained Dialogflow model. It also extracts entity data and makes that available on flow
scope. Read the Dialogflow docs
This component requires a Dialogflow v2 agent and the Dialogflow integration. If you are using a v1 agent, upgrade your agent or use the
meya.api_ai
component.
Property | Description | |
---|---|---|
text | The text to output to the user | Required |
speech | Text to speak to the user. This field also accepts SSML markup to customize pronunciation. | Optional |
project_id | Found in your Dialogflow agent's settings. | Required |
require_match | If false the flow will return an action no_match that you can use to transition to another state | Optional. Default: true |
min_confidence | The minimum confidence required to match the entity. | Optional. Default: 0.67 |
language | Two letter language code. ex "en" matching the api.ai agent's language. If omitted, the bot will set flow.language to the language the input was written in. | Optional. Default: blank (i.e. auto-detect) |
scope | Where to store the intent + entity data. One of flow , user , bot . | Optional. Default: flow |
latch_up | If true , any confidence value greater than min_confidence will be round up to 1.0 , thus winning any intent collisions. Confidence values less than min_confidence will be rounded down to 0.0 . | Optional. Default: false |
buttons | an array of Buttons | Optional |
cache_duration | The number of seconds a request is cached. Set to 0 to force a new request. Note that shorter cache durations may increase latency in bot response since more API calls must be made. | Optional. Default: 60 |
Transitions
next: the default transition for an answer (if not present, flow will transition to the next state in sequence)
no_match: the state to transition to if require_match
is false
and the user inputs something ambiguous
The captured user intent will be available as
flow.intent
later in the next step(s) of your flow.
dialogflow_state:
component: meya.dialogflow
properties:
text: "How are you doing today?"
project_id: XXXX
language: en
error_message: "I don't understand"
min_confidence: 0.8
require_match: false
transitions:
bad: bad_state
good: good_state
no_match: ambiguous_state
bad_state:
component: meya.text
properties:
text: "That's too bad."
return: true
good_state:
component: meya.text
properties:
text: "Great!"
return: true
meya.wit
Accepts input from the user and matches it against a pre-trained wit.ai model. It also extracts entity data and makes that available on flow
scope. Read the wit docs
Categorizing the user intent
If you are going to use the
transitions
property of this component you should train your model to classify utterances using theintent
entity that categorizes the user intent. See example
Property | Description | |
---|---|---|
text | The text to output to the user | Required |
speech | Text to speak to the user. This field also accepts SSML markup to customize pronunciation. | Optional |
token | The wit.ai secret server token for your wit.ai app settings | Required |
require_match | If false the flow will return an action no_match that you can use to transition to another state | Optional. Default: true |
min_confidence | The minimum confidence required to match the entity. | Optional. Default: 0.67 |
latch_up | If true , any confidence value greater than min_confidence will be round up to 1.0 , thus winning any intent collisions. Confidence values less than min_confidence will be rounded down to 0.0 . | Optional. Default: false |
scope | Where to store the intent + entity data. One of flow, user, bot. | Optional. Default: flow |
buttons | An array of Buttons | Optional |
cache_duration | The number of seconds a request is cached. Set to 0 to force a new request. Note that shorter cache durations may increase latency in bot response since more API calls must be made. | Optional. Default: 60 |
Transitions
next: the default transition for an answer (if not present, flow will transition to the next state in sequence)
no_match: the state to transition to if require_match
is false
and the user inputs something ambiguous
The captured user intent will be available as
flow.intent
later in the next step(s) of your flow.
component: meya.wit
properties:
text: "How do you feel?"
token: XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
error_message: "I don't understand"
min_confidence: 0.8
require_match: false
transitions:
bad: bad_state
good: good_state
no_match: ambiguous_state
meya.api_ai
Accepts input from the user and matches it against a pre-trained api.ai model. It also extracts entity data and makes that available on flow
scope. Read the api.ai docs
This component does not support Dialogflow v2 features, such as multi-language agents. If you wish to make use of those features, use the
meya.dialogflow
component with your v2 agent.
Property | Description | |
---|---|---|
text | the text to output to the user | |
speech | Text to speak to the user. This field also accepts SSML markup to customize pronunciation. | Optional |
client_access_token | The api.ai client access token from your api.ai agent settings | Required |
language | Two letter language code. ex "en" matching the api.ai agent's language. If omitted, the bot will set flow.language to the language the input was written in. | Optional. Default: blank (i.e. auto-detect) |
require_match | If false the flow will return an action no_match that you can use to transition to another state | Optional. Default: true |
min_confidence | the minimum confidence to match the entity | Optional. Default: 0.67 |
latch_up | If true , any confidence value greater than min_confidence will be round up to 1.0 , thus winning any intent collisions. Confidence values less than min_confidence will be rounded down to 0.0 . | Optional. Default: false |
scope | where to store the intent + entity data. One of flow, user, bot. | Optional. Default: flow |
buttons | an array of Buttons | Optional |
cache_duration | The number of seconds a request is cached. Set to 0 to force a new request. Note that shorter cache durations may increase latency in bot response since more API calls must be made. | Optional. Default: 60 |
Transitions
next: the default transition for an answer (if not present, flow will transition to the next state in sequence)
no_match: the state to transition to if require_match
is false
and the user inputs something ambiguous
The captured user intent will be available as
flow.intent
later in the next step(s) of your flow.
component: meya.api_ai
properties:
text: "How do you feel?"
client_access_token: XXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXXX
language: en
error_message: "I don't understand"
min_confidence: 0.8
require_match: false
transitions:
bad: bad_state
good: good_state
no_match: ambiguous_state
Intents are used to transition
In the
transitions
section of the code snippet above, bothbad
andgood
must be intents you've created in your Dialogflow agent. If they don't exist, this code snippet will not work.Similarly, any Dialogflow intent you want to handle in this component must have an entry in the
transitions
section, otherwise nothing will happen when the user expresses that intent.
Example - Language Detection
You can use the meya.api_ai
component to detect a user's language. Start by creating a Dialogflow agent with both English and French language enabled. Then create a greetings
intent in the agent with English and French training phrases, such as hello
and bonjour
. Finally, create a new flow called language
and paste the following code.
states:
first:
component: meya.text
properties:
text: "Your language is {{ flow.language }}"
api_ai_state:
component: meya.api_ai
properties:
text: "Enter some text"
client_access_token: <YOUR_CLIENT_ACCESS_TOKEN>
transitions:
greeting: greeting
greeting:
component: meya.text
properties:
text: "Your language is {{ flow.language }}"
Test it out in the test chat window. Enter hello
and the bot will respond Your language is en
. Enter bonjour
and the bot will say Your language is fr
.
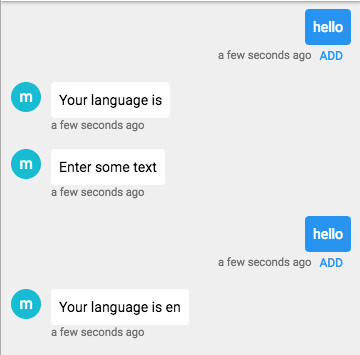
meya.luis
Accepts input from the user and matches it against a pre-trained luis.ai model. It also extracts entity data and makes that available on flow
scope. Read the luis docs
Property | Description | |
---|---|---|
text | the text to output to the user | |
speech | Text to speak to the user. This field also accepts SSML markup to customize pronunciation. | Optional |
app_id | luis.ai app id | Required |
api_key | luis.ai api key | Required |
subdomain | Within your Luis app, you can specify which geographic regions your app will be available in. Within Meya, you can specify which one you're using by setting subdomain . | Optional. Default: westus |
require_match | If false the flow will return an action no_match that you can use to transition to another state | Optional. Default: true |
min_confidence | the minimum confidence to match the entity | Optional. Default: 0.67 |
latch_up | If true , any confidence value greater than min_confidence will be round up to 1.0 , thus winning any intent collisions. Confidence values less than min_confidence will be rounded down to 0.0 . | Optional. Default: false |
scope | where to store the intent + entity data. One of flow, user, bot. | Optional. Default: flow |
buttons | an array of Buttons | Optional |
cache_duration | The number of seconds a request is cached. Set to 0 to force a new request. Note that shorter cache durations may increase latency in bot response since more API calls must be made. | Optional. Default: 60 |
Transitions
next: the default transition for an answer (if not present, flow will transition to the next state in sequence)
no_match: the state to transition to if require_match
is false
and the user inputs something ambiguous
The captured user intent will be available as
flow.intent
later in the next step(s) of your flow.
component: meya.luis
properties:
text: "How do you feel?"
app_id: xxxxxxxxxxxxxxxxxxxxxxxxxx
api_key: xxxxxxxxxxxxxxxxxxxxxxxxxx
error_message: "I don't understand"
min_confidence: 0.8
transitions:
bad: bad_state
good: good_state
Intents are used to transition
In the
transitions
section of the code snippet above, bothfinancing
andinsurance
must be intents you've created in your Luis agent. If they don't exist, this code snippet will not work.Similarly, any Luis intent you want to handle in this component must have an entry in the
transitions
section, otherwise nothing will happen when the user expresses that intent.
meya.nlp_yes_no
Accepts input from the user and matches it against a pre-trained yes/no model (English).
Property | Description | |
---|---|---|
text | the text to output to the user | |
speech | Text to speak to the user. This field also accepts SSML markup to customize pronunciation. | Optional |
require_match | If false the flow will return an action no_match that you can use to transition to another state | Optional. Default: true |
Transitions
next: the default transition for an answer (if not present, flow will transition to the next state in sequence)
no_match: the state to transition to if require_match
is false
and the user inputs something ambiguous
yes
andno
are reserved in YAML so must be escaped with single or double quotes.
component: meya.nlp_yes_no
properties:
text: Do you like Meya?
transitions:
"yes": yes_state
"no": no_state
Updated about 6 years ago