Components
The unit of work.
Components are units of work within a flow. You can either use built-in components like meya.text
or write your own custom component in Python. A full list of core components can be found here.
component: {component_name}
properties:
{key1}: {value1}
{key2}: {value2}
...
transitions:
{action1}: {state1}
{action2}: {state2}
...
By default flows return the
next
action which is not defined in thetransitions
section, the flow will automatically and advance to the next state.
Returning multiple messages
A custom component can return multiple messages (up to 5). The messages can be of different types (e.g. plain text, card with buttons).
from meya import Component
class HelloWorld(Component):
def start(self):
text = "Hello, world!"
message = self.create_message(text=text, speech=text)
return self.respond(message=message, action="next")
from meya import Component
from meya.cards import Image
class MultipleMessages(Component):
def start(self):
messages = []
card = Image(image_url="http://i.imgur.com/0F374vh.jpg")
messages.append(self.create_message(card=card))
text = "All done!"
messages.append(self.create_message(text=text))
return self.respond(messages=messages, action='next')
speech
only needs to be set if you're using a voice integration.
Maximum number of messages per state
Each state can generate a maximum of 5 messages. If your component returns more than 5 messages, the additional messages will be discarded. If you find messages are not being delivered to the user, verify that you are not exceeding this limit.
Cloud deployment
Components are run in an isolated cloud environment. When you click Save, Meya will deploy the code to its own runtime environment in which your code can access core elements of the Meya Bot Framework. For example, you can access Meya's built-in datastore and code dependencies like Python's requests
.
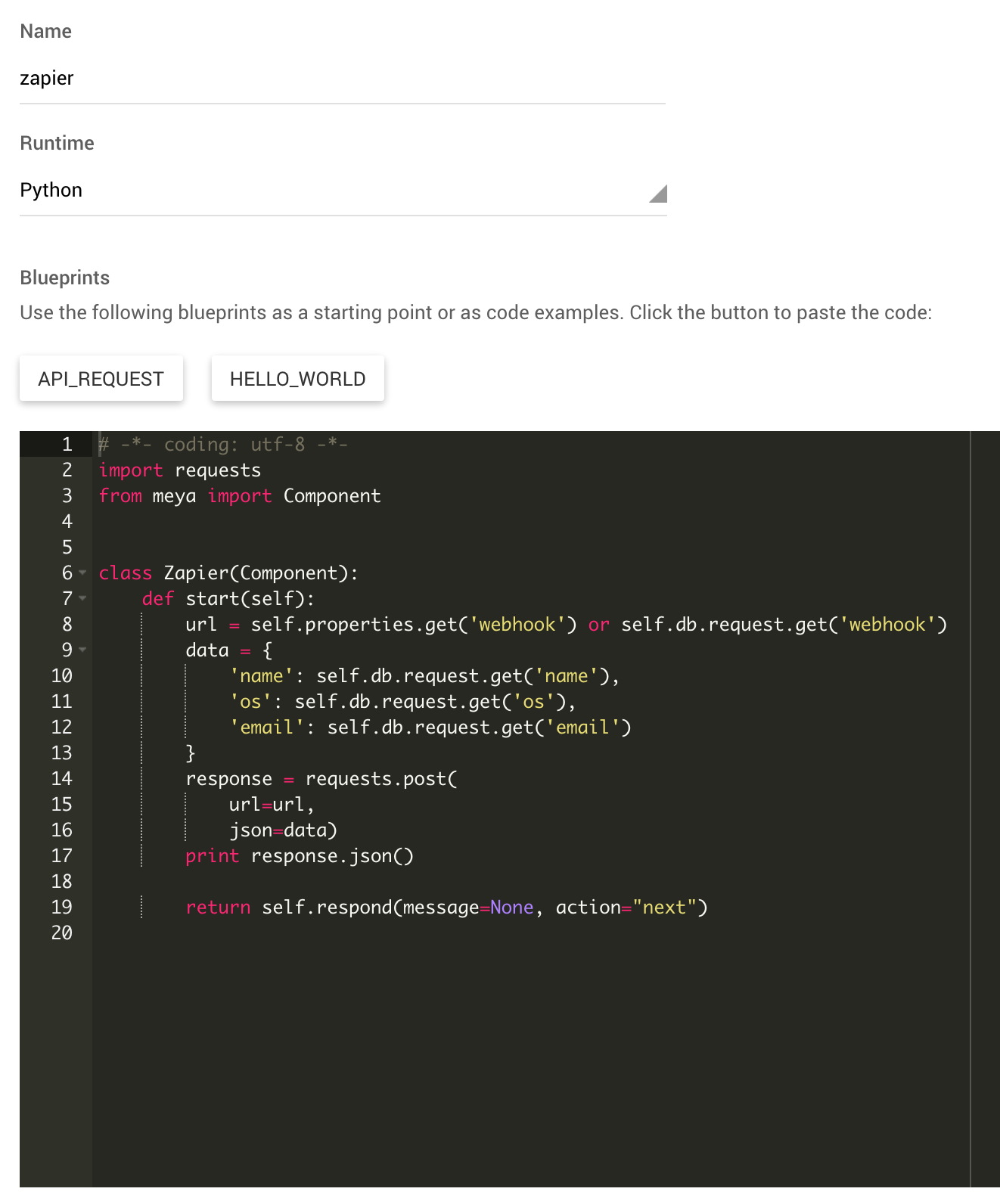
Example Python component.
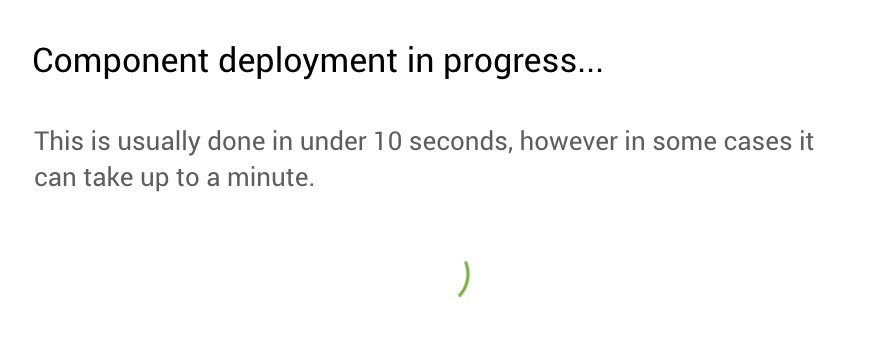
Component is deploying to cloud.
Testing
The web environment provides a component test facility that allows you to unit test your component to ensure that it works within the context of the flow.
{
"bot": {},
"flow": {
"os": "Android",
"webhook": "https://zapier.com/hooks/catch/532172/u2b7em/",
"name": "Jack Johnson",
"email": "[email protected]"
},
"user": {}
}
You can provide custom test data using JSON to simulate bot context.
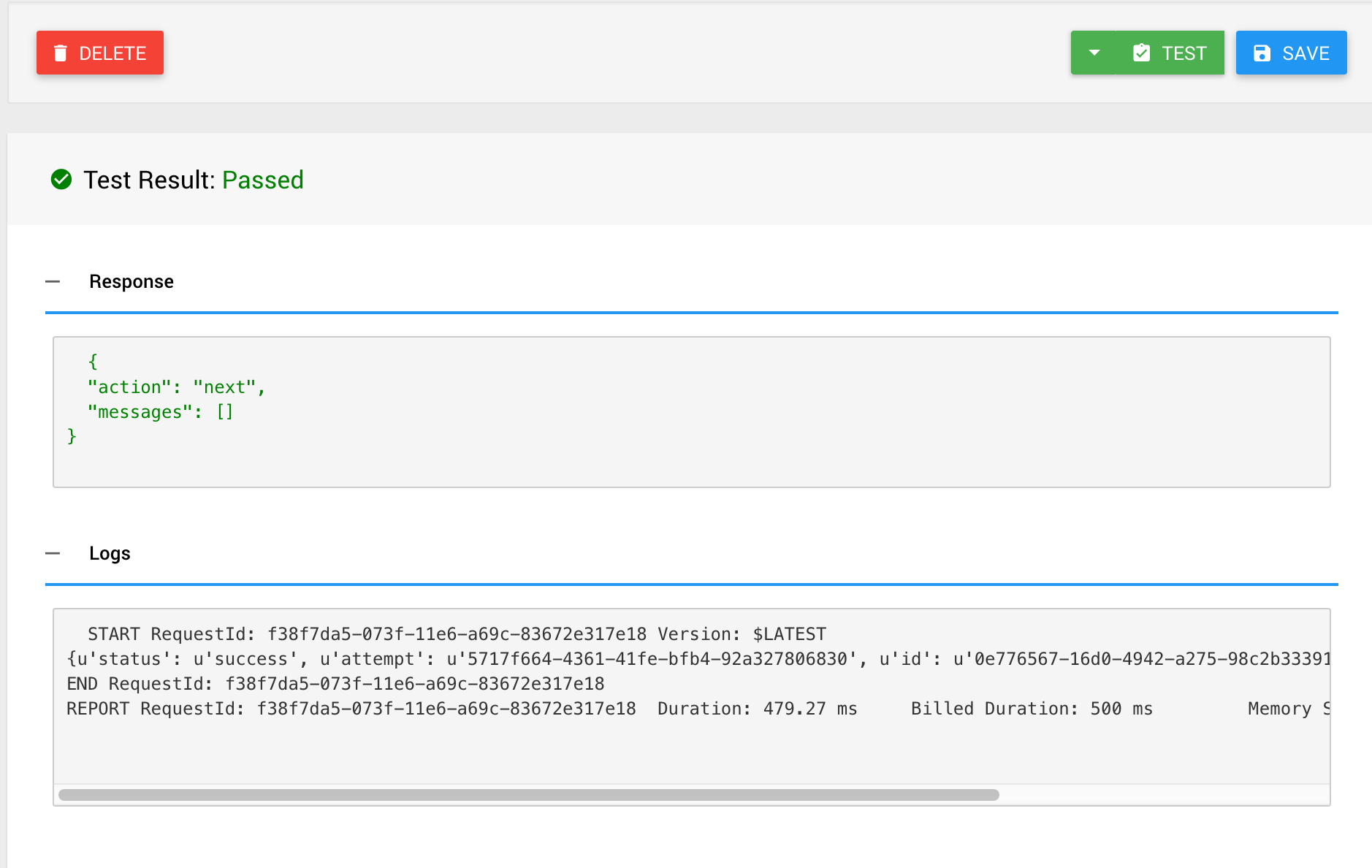
Response and log output of running a test.
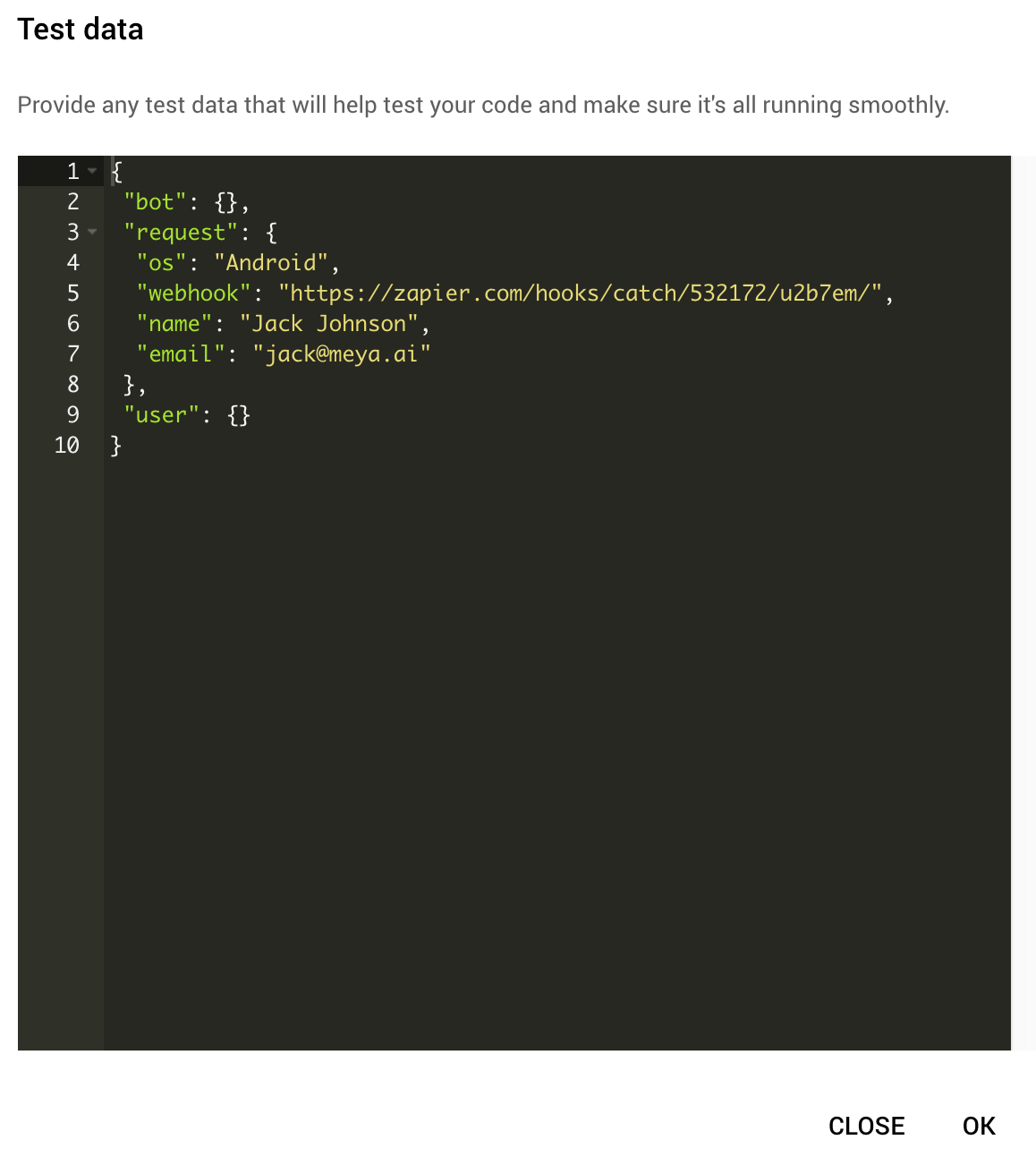
Specify test data
Updated over 4 years ago