Generic cards
Image and/or text with optional carousel
meya.card
A comprehensive card that contains a mix of text and images. Renders on each integration differently, depending on the specifications of the integration (e.g. on Messenger suggestions are buttons, on Kik they are keyboard suggested responses). Read about the multi-bubble card Messenger components
Property | Description | |
---|---|---|
| The title of the card | Required |
| The text to output | Messenger - this is the subtitle (limit 80 characters) |
| The url of the image to output | |
| Aspect ratio used to render the image from the image_url. Must be |
|
| A list of suggested responses | or buttons |
| An array of Buttons | or suggestions |
| Automatically advance without waiting on user |
|
| Treat any non-button press as a user input. This will trigger the |
|
| The key used to store the data for subsequent steps in the flow |
|
| Where to store the data. One of flow, user, bot |
|
| A web URL that is invoked when the user clicks on the card. | Optional Deprecated |
| A description of the image. |
|
| A one-line string. Extra characters are truncated. | Actions on Google only. |
| One of | Actions on Google only. Default: |
| This property is only valid when the card is part of a card carousel ( |
|
*Messenger only field
Example of a card with suggestions
used in a flow and a custom component.
component: meya.card
properties:
title: "Elephants are big"
text: "They are really big sometimes."
image_url: "http://bit.ly/1NPO0xx"
suggestions:
- Agreed
- Whales are bigger
from meya.cards import Card, Button
title = "Elephants are big"
text = "They are really big sometimes."
image_url = "http://bit.ly/1NPO0xx"
buttons = [
Button(text='Agreed'),
Button(text='Whales are bigger')
]
card = Card(title=title,
text=text,
image_url=image_url,
buttons=buttons)
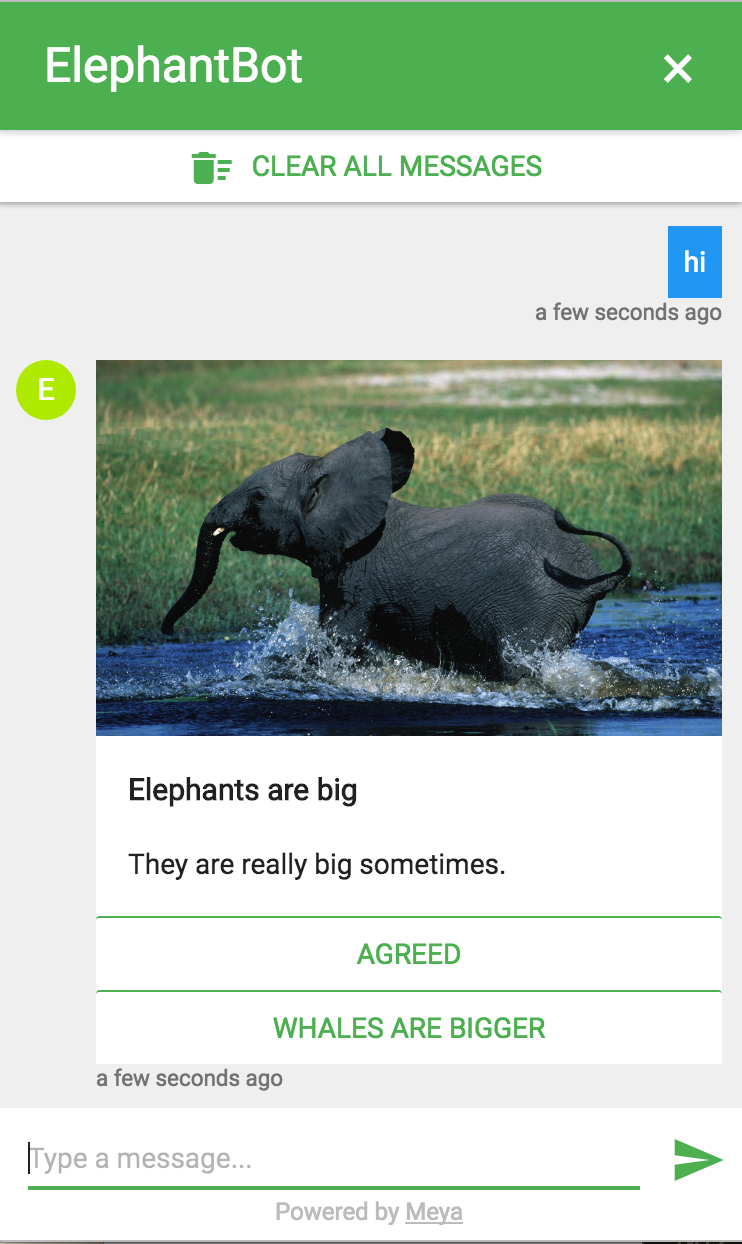
Example of card with buttons
used in a flow.
component: meya.card
properties:
title: "Which is your favorite elephant?"
text: "All of them are pretty cool."
image_url: "http://bit.ly/1NPO0xx"
buttons:
- text: "African Elephant"
action: vote_result
data:
vote: african
- text: "Asian Elephant"
action: vote_result
data:
vote: asian
- text: "Woolly Mammoth"
action: vote_result
data:
vote: woolly
from meya.cards import Card, Button
title = "Which is your favorite elephant?"
text = "All of them are pretty cool."
image_url = "http://bit.ly/1NPO0xx"
buttons = [
Button(text='African Elephant',
action='vote_result',
data={'vote': 'african'}),
Button(text='Asian Elephant',
action='vote_result',
data={'vote': 'asian'}),
Button(text='Woolly Mammoth',
action='vote_result',
data={'vote': 'woolly'})
]
card = Card(title=title,
text=text,
image_url=image_url,
buttons=buttons)
Special considerations for Actions on Google
If you wish to display a card without any buttons, you'll need to ensure passthru
is true
and expect_user_action
is false
. See the Without buttons code example below.
If you do not specify image_url
you must specify text
. Likewise, if you do not specify text
you must specify image_url
.
The text
property cannot contain a URL.
A card may have at most one link button. Without a link button, the card will have no interaction capabilities.
Here are some examples:
component: meya.card
properties:
title: "Which is your favorite elephant?"
subtitle: "Choose an options below."
text: "All of them are pretty cool."
image_url: "http://bit.ly/1NPO0xx"
accessibility_text: "A picture of an elephant"
image_display_options: CROPPED
buttons:
- text: "African Elephant"
action: vote_result
data:
vote: african
- text: "Asian Elephant"
action: vote_result
data:
vote: asian
- text: "See more pictures of elephants"
url: "https://example.com"
component: meya.card
properties:
title: "Here's a picture of an elephant."
subtitle: "Isn't it cool?"
text: "Actually, all elephants are cool."
image_url: "http://bit.ly/1NPO0xx"
accessibility_text: "A picture of an elephant"
image_display_options: CROPPED
passthru: true
expect_user_action: false
meya.cards
A multi-bubble card that allows you to output multiple cards in a single message. Note: it is best practice to use buttons in this case, not suggestions.
Support
Currently only Messenger, Meya Web, and Actions on Google support the multi-bubble card.
Property | Description | |
---|---|---|
| An array of cards | Required |
| Aspect ratio used to render all the images of the elements. Must be |
|
| Automatically advance without waiting on user |
|
| Treat any non-button press as a user input. This will trigger the |
|
| The key used to store the data for subsequent steps in the flow |
|
| Where to store the data. One of flow, user, bot |
|
Example of a multi-bubble card using all 3 button types. Each element uses 3 different types of buttons - #1 link button, #2 - transition button, #3 - start button.
component: meya.cards
properties:
output: button_click
image_aspect_ratio: square
elements:
- title: African Elephant
text: "Elephants are extremely big"
item_url: "https://en.wikipedia.org/wiki/African_elephant"
image_url: "http://i.imgur.com/7Z9IX5W.jpg"
buttons:
- text: See Wikipedia page
url: "https://en.wikipedia.org/wiki/African_elephant"
- text: See more
action: next
- text: Vote
flow: vote_result
data:
vote: african
- title: Asian Elephant
text: "In general, the Asian elephant is smaller than the African elephant."
item_url: "https://en.wikipedia.org/wiki/Asian_elephant"
image_url: "http://i.imgur.com/w5qzZ7I.jpg"
buttons:
- text: See Wikipedia page
url: "https://en.wikipedia.org/wiki/Asian_elephant"
- text: See more
action: next
- text: Vote
flow: vote_result
data:
vote: asian
from meya.cards import Card, Cards, Button
buttons = [
Button(
text='See Wikipedia page',
url='https://en.wikipedia.org/wiki/African_elephant'
),
Button(
text='See more',
action='next'
),
Button(
text='Vote',
flow='vote_result',
data={'vote': 'african'}
)
]
element1 = Card(
title='African Elephant',
text='One species of African elephant is the largest living terrestrial animal.',
item_url='https://en.wikipedia.org/wiki/African_elephant',
image_url='http://i.imgur.com/7Z9IX5W.jpg',
buttons=buttons)
buttons = [
Button(
text='See Wikipedia page',
url='https://en.wikipedia.org/wiki/Asian_elephant'
),
Button(
text='See more',
action='next'
),
Button(
text='Vote',
flow='vote_result',
data={'vote': 'asian'}
)
]
element2 = Card(
title='African Elephant',
text='In general, the Asian elephant is smaller than the African elephant.',
item_url='https://en.wikipedia.org/wiki/Asian_elephant',
image_url='http://i.imgur.com/w5qzZ7I.jpg',
buttons=buttons)
card = Cards(elements=[element1, element2])
Special considerations for Actions on Google
All cards in the carousel must have the same components. For example, if one card has an image, all of the cards must have an image.
If you wish to display cards without any buttons, you'll need to ensure passthru
is true
and expect_user_action
is false
. See the Without buttons code example below.
component: meya.cards
properties:
output: button_click
image_aspect_ratio: square
elements:
- title: "African Elephant"
text: "Elephants are extremely big"
item_url: "https://en.wikipedia.org/wiki/African_elephant"
image_url: "http://i.imgur.com/7Z9IX5W.jpg"
accessibility_text: "An African elephant"
footer: "This is some footer text"
buttons:
- text: "See Wikipedia page"
url: "https://en.wikipedia.org/wiki/African_elephant"
- text: "See more"
action: next
- text: "Vote"
flow: vote_result
data:
vote: african
- title: "Asian Elephant"
text: "In general, the Asian elephant is smaller than the African elephant."
item_url: "https://en.wikipedia.org/wiki/Asian_elephant"
image_url: "http://i.imgur.com/w5qzZ7I.jpg"
accessibility_text: "An Asian elephant"
footer: "This is some footer text"
buttons:
- text: "See Wikipedia page"
url: "https://en.wikipedia.org/wiki/Asian_elephant"
- text: "See more"
action: next
- text: "Vote"
flow: vote_result
data:
vote: asian
Updated 18 days ago