Buttons
buttons
and suggestions
are a great way to transition through your flow. They are rendered differently depending on the integration.
Suggestions are suggested responses that you can output to the user. When the user selects a suggestion, it comes in as if the user typed the text.
Buttons are a more advanced way to transition through the flow, link to a webpage or start a new flow.
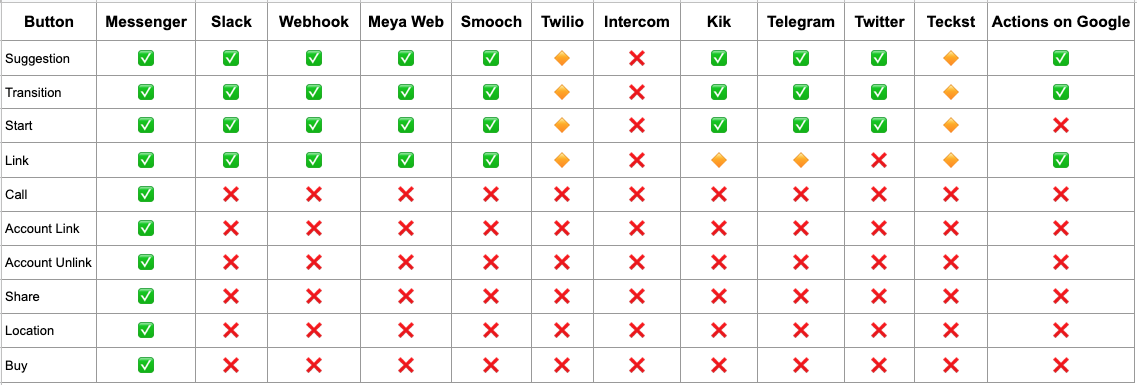
Button support matrix
Suggestion
When clicked, it's as if the user typed the text. See the button support matrix for the integrations that support suggestions. Suggestions are rendered on Messenger as quick replies.
suggestions:
- African Elephant
- Asian Elephant
- Woolly Mammoth
Buttons
There are 3 main types buttons: transition
buttons, link
buttons and start
buttons. Transitions move you through the flow, links are links to webpages and start buttons start new flows.
There are 4 more buttons available only on Facebook Messenger: account_link
, account_unlink
, share
and location
(rendered as a quick_reply).
Transition button
Moves you through the flow. See the button support matrix for the integrations that support transition buttons.
Parameters | Description | |
---|---|---|
text | the button text | Required |
action | the action fired on button click | Optional. Default: next |
data | the data saved on button click | Optional. Saved to the datastore |
A Suggestion is really a special case of a Transition Button with only the text set.
Example of transition buttons using the same action (vote_result
) regardless of what is clicked and saving data to the flow.
data: the vote
data is accessed through flow.vote
buttons:
- text: African Elephant
action: vote_result
data:
vote: african
from meya.cards import Button
button = Button(
text="African Elephant",
action="vote_result",
data={'vote': "african"}
)
Example of a transition button that traverses through the flow depending on which button is clicked.
states:
text_buttons_state:
component: meya.text_buttons
properties:
text: Pick your favorite football player
output: player
buttons:
- text: Lionel Messi
action: messi
- text: Cristiano Ronaldo
action: ronaldo
transitions:
messi: messi_state
ronaldo: ronaldo_state
messi_state:
component: meya.image
properties:
image_url: 'https://upload.wikimedia.org/wikipedia/commons/f/f2/Lionel_Messi.png'
return: true
ronaldo_state:
component: meya.image
properties:
image_url: 'https://upload.wikimedia.org/wikipedia/commons/d/d5/Cristiano_Ronaldo_20120609.jpg'
return: true
Note
Once a transition button has been clicked, the remaining buttons in the card will no longer work.
Start button
Starts a new flow. See the button support matrix for the integrations that support start buttons.
Parameters | Description | |
---|---|---|
text | the button text | Required |
flow | the name of the flow to transition to | Required. Flow must exist |
data | the data saved on button click and passed to the flow | Optional |
action | used as the intent to infer which state to start with | Optional. Default: start |
Example of a button click that will start a new flow elephant_info
. The elephant type will be passed through the flow as flow.favorite
buttons:
- text: African Elephant
flow: elephant_info
data:
favorite: african
from meya.cards import Button
button = Button(
text="African Elephant",
flow="elephant_info",
data={'favorite': "african"}
)
Link button
Links to a webpage. See the button support matrix for the integrations that support link buttons.
A link button can also be used for Facebook Messenger's Call button, simply set the link to
tel://phone_number
Parameter | Description | |
---|---|---|
text | the button text | Required |
url | the link to open. This can also be a phone number tel://5551234567 or deep link appster://item/view?id=1234 | Required |
webview_height_ratio | compact , tall or full | optional For Facebook Messenger and Smooch Web Messenger |
fallback_url | URL to use on clients that don't support Messenger Extensions. Defaults to the value of url . | optional For Facebook Messenger and Smooch Web Messenger |
messenger_extensions | set to true to use Messenger Extensions | optional For Facebook Messenger |
Example of a link button sending the user to a Wikipedia page.
buttons:
- text: African Elephant
url: "https://en.wikipedia.org/wiki/African_bush_elephant"
from meya.cards import Button
button = Button(
text="African Elephant",
url="https://en.wikipedia.org/wiki/African_bush_elephant"
)
Buy button
Accept payments with Facebook Messenger.
Account link button
Allows you to connect your user with a Messenger user. Upon a successful account linking, an authorization_code
will be saved to the user.
The button text is set automatically by Facebook to Log In.
Parameter | Description | |
---|---|---|
type | Must be set to account_link | Required |
url | a webpage where the user can login to your service. If account linking is successful, redirect the browser to the redirect_uri specified in your callback to complete the linking flow, and append the authorization_code parameter | Required |
action | the action fired on button click |
buttons:
- type: account_link
url: "https://www.example.com/oauth/authorize"
action: login
from meya.cards import Button
button = Button(
type="account_link",
url="https://www.example.com/oauth/authorize",
action="login"
)
Account unlink button
Allows Messenger users to logout of your service.
The button text is set automatically by Facebook to Log Out.
Property | Description | |
---|---|---|
type | account_unlink | Required |
buttons:
- type: account_unlink
action: logout
from meya.cards import Button
button = Button(type="account_unlink", action="logout")
Share button
Allows people to share a card message to other Messenger users.
The button text is set automatically by Facebook to Share.
Only supported by Facebook Messenger. See full docs
Property | Description | |
---|---|---|
type | share | Required |
buttons:
- type: share
from meya.cards import Button
button = Button(type="share")
The share_contents property
Facebook allows you to modify the Share button so users can add a custom message to the link when they share it. By setting the share_contents
property, you can also determine how the content will look when it's shared, including setting a button name (instead of the default value of Share).
Here's an example of a Share button that uses the share_contents
property.
states:
first:
component: meya.image_buttons
properties:
image_url: "https://apricot.blender.org/wp-content/uploads/2008/10/yofrankie10.jpg?x20393"
buttons:
- type: share
share_contents:
attachment:
type: "template"
payload:
template_type: "generic"
elements:
- title: "I took Peter's 'Which Hat Are You?' Quiz"
subtitle: "My result: Fez"
image_url: "https://www.villagehatshop.com/photos/product/standard/4511390S91097/all/c-crown-crushable-wool-felt-fedora-hat.jpg"
default_action:
type: "web_url"
url: "https://www.villagehatshop.com/hats/Wide-Brim-Fedora"
buttons:
- type: "web_url"
url: "https://www.villagehatshop.com/hats/Wide-Brim-Fedora"
title: "Check These Hats"
Location quick reply
Allows you to prompt a person to share their location.
The buttons must be in
quick_reply
mode.
Only supported by Facebook Messenger.
Property | Description | |
---|---|---|
type | location | Required |
action | the action fired on button click | Optional. If set, the button will behave as transition button, otherwise, the button will fire any location start intents to new flows |
states:
location:
component: meya.text_buttons
properties:
text: Where are you?
output: button_click
scope: flow
mode: quick_reply
buttons:
- type: location
action: sendlocation
- text: No, thanks
action: nope
transitions:
nope: done
sendlocation: location_out
location_out:
component: meya.text
properties:
text: "🗺 You are here: {{ flow.lat }}, {{ flow.lng }}"
location_out2:
component: meya.text
properties:
text: "How is the weather in {{ flow.city }}?"
return: true
done:
component: meya.text
properties:
text: "You're entitled to your privacy."
return: true
from meya.cards import Button
button = Button(type="share", text="Share my location", action="next")
The location data will be stored in the specified scope
, and behaves similarly to the meya.input_location
component (see docs).
Paramater | Type |
---|---|
lat | float |
lng | float |
timezone | string |
city | string |
state | string |
country | string |
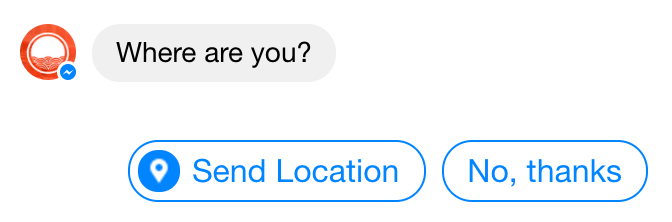
What the send location button looks like
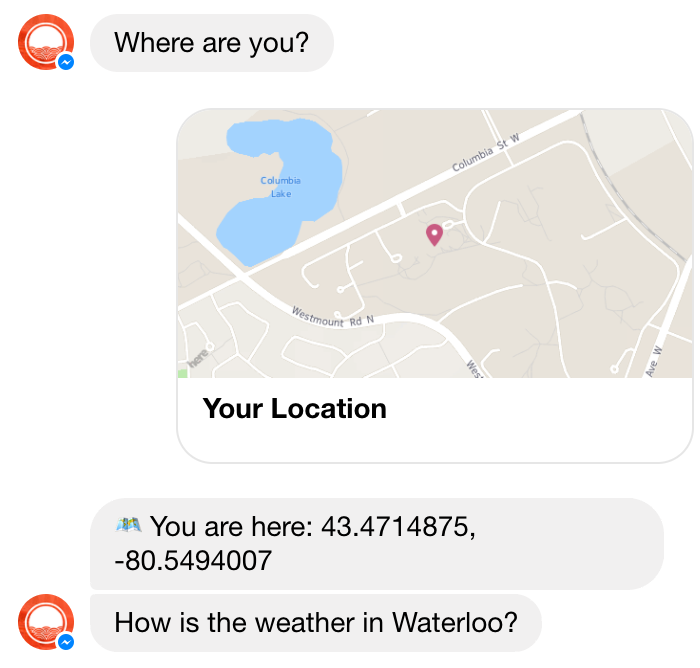
What you can do after the user has sent location
Updated almost 4 years ago