Sharing custom components
Shared components
You can import custom components into other custom components using the following syntax:
import components.<component_name>
You could use this feature to create a custom component that holds functions your other custom components will share. First, create a component called utilities.py
.
def hello_world():
print "Hello, world!"
def square(x):
return x*x
Then create a custom component called test.py
that uses the functions in utilities.py
.
from meya import Component
from components.utilities import hello_world, square
class First(Component):
def start(self):
hello_world()
print square(5)
return self.respond(message=None, action="next")
Finally, add a flow that uses the test.py
component. Set the trigger to catchall
.
states:
start:
component: test
transitions:
next: success
success:
component: meya.pass
In the test chat on the right side of the Bot Studio, submit any text. This will trigger the flow.
In the debug console, you should see the results printed by test.py
.
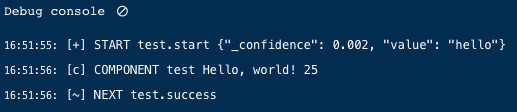
Updated over 6 years ago