Airline cards
Cards that are specific to airline-related information
Boarding Pass Template
Send a message that contains boarding passes for one or more flights or one more passengers. Message bubbles will be grouped by flight information -- if the flight information matches, all passengers will be share the same bubble. Multiple bubbles are automatically sent for all boarding_pass elements with different values for flight_info. In the future, Facebook may group all boarding passes into the same bubble.
See Facebook's documentation here
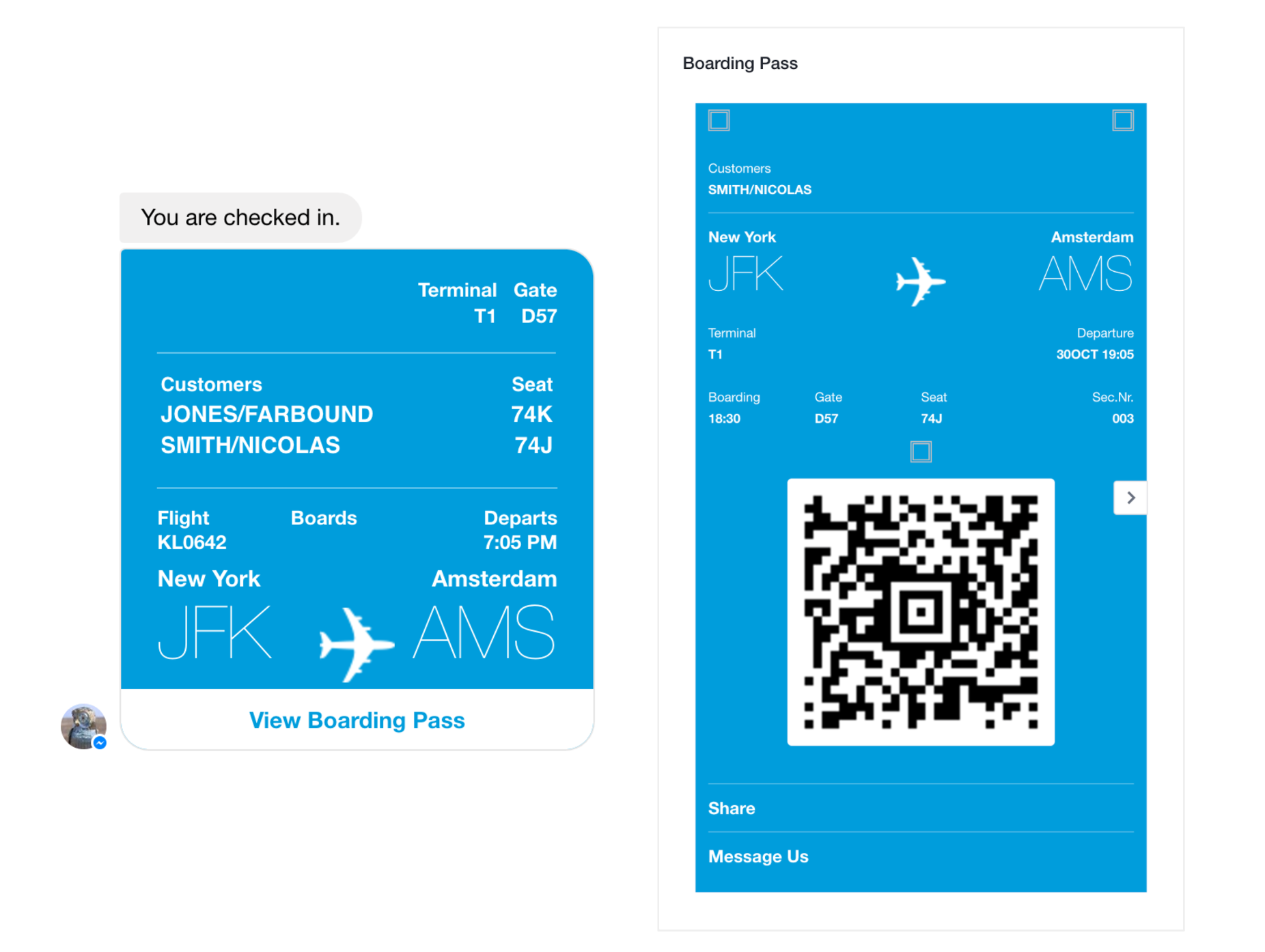
Boarding pass message on left. Boarding pass pop-up on right (after clicking "View Boarding Pass").
component: meya.airline_boardingpass
properties:
intro_message: You are checked in.
locale: en_US
boarding_pass:
- passenger_name: SMITH/NICOLAS
pnr_number: CG4X7U
travel_class: business
seat: 74J
auxiliary_fields:
- label: Terminal
value: T1
- label: Departure
value: 30OCT 19:05
secondary_fields:
- label: Boarding
value: '18:30'
- label: Gate
value: D57
- label: Seat
value: 74J
- label: Sec.Nr.
value: '003'
logo_image_url: https://www.example.com/en/logo.png
header_image_url: https://www.example.com/en/fb/header.png
qr_code: M1SMITH/NICOLAS CG4X7U nawouehgawgnapwi3jfa0wfh
above_bar_code_image_url: https://www.example.com/en/PLAT.png
flight_info:
flight_number: KL0642
departure_airport:
airport_code: JFK
city: New York
terminal: T1
gate: D57
arrival_airport:
airport_code: AMS
city: Amsterdam
flight_schedule:
departure_time: 2016-01-02T19:05
arrival_time: 2016-01-05T17:30
- passenger_name: JONES/FARBOUND
pnr_number: CG4X7U
travel_class: business
seat: 74K
auxiliary_fields:
- label: Terminal
value: T1
- label: Departure
value: 30OCT 19:05
secondary_fields:
- label: Boarding
value: '18:30'
- label: Gate
value: D57
- label: Seat
value: 74K
- label: Sec.Nr.
value: '004'
logo_image_url: https://www.example.com/en/logo.png
header_image_url: https://www.example.com/en/fb/header.png
qr_code: M1JONES/FARBOUND CG4X7U nawouehgawgnapwi3jfa0wfh
above_bar_code_image_url: https://www.example.com/en/PLAT.png
flight_info:
flight_number: KL0642
departure_airport:
airport_code: JFK
city: New York
terminal: T1
gate: D57
arrival_airport:
airport_code: AMS
city: Amsterdam
flight_schedule:
departure_time: 2016-01-02T19:05
arrival_time: 2016-01-05T17:30
from meyacards import AirlineBoardingPass
BOARDING_PASS = {
"intro_message": "You are checked in.",
"locale": "en_US",
"boarding_pass": [
{
"passenger_name": "SMITH\/NICOLAS",
"pnr_number": "CG4X7U",
"travel_class": "business",
"seat": "74J",
"auxiliary_fields": [
{
"label": "Terminal",
"value": "T1"
},
{
"label": "Departure",
"value": "30OCT 19:05"
}
],
"secondary_fields": [
{
"label": "Boarding",
"value": "18:30"
},
{
"label": "Gate",
"value": "D57"
},
{
"label": "Seat",
"value": "74J"
},
{
"label": "Sec.Nr.",
"value": "003"
}
],
"logo_image_url": "https://www.example.com/en/logo.png",
"header_image_url": "https://www.example.com/en/fb/header.png",
"qr_code": "M1SMITH\/NICOLAS CG4X7U nawouehgawgnapwi3jfa0wfh",
"above_bar_code_image_url": "https://www.example.com/en/PLAT.png",
"flight_info": {
"flight_number": "KL0642",
"departure_airport": {
"airport_code": "JFK",
"city": "New York",
"terminal": "T1",
"gate": "D57"
},
"arrival_airport": {
"airport_code": "AMS",
"city": "Amsterdam"
},
"flight_schedule": {
"departure_time": "2016-01-02T19:05",
"arrival_time": "2016-01-05T17:30"
}
}
},
{
"passenger_name": "JONES\/FARBOUND",
"pnr_number": "CG4X7U",
"travel_class": "business",
"seat": "74K",
"auxiliary_fields": [
{
"label": "Terminal",
"value": "T1"
},
{
"label": "Departure",
"value": "30OCT 19:05"
}
],
"secondary_fields": [
{
"label": "Boarding",
"value": "18:30"
},
{
"label": "Gate",
"value": "D57"
},
{
"label": "Seat",
"value": "74K"
},
{
"label": "Sec.Nr.",
"value": "004"
}
],
"logo_image_url": "https://www.example.com/en/logo.png",
"header_image_url": "https://www.example.com/en/fb/header.png",
"qr_code": "M1JONES\/FARBOUND CG4X7U nawouehgawgnapwi3jfa0wfh",
"above_bar_code_image_url": "https://www.example.com/en/PLAT.png",
"flight_info": {
"flight_number": "KL0642",
"departure_airport": {
"airport_code": "JFK",
"city": "New York",
"terminal": "T1",
"gate": "D57"
},
"arrival_airport": {
"airport_code": "AMS",
"city": "Amsterdam"
},
"flight_schedule": {
"departure_time": "2016-01-02T19:05",
"arrival_time": "2016-01-05T17:30"
}
}
}
]
}
boarding_pass_card = AirlineBoardingPass(payload=BOARDING_PASS)
Airline Checkin Template
A check-in reminder message.
See Facebook's documentation here
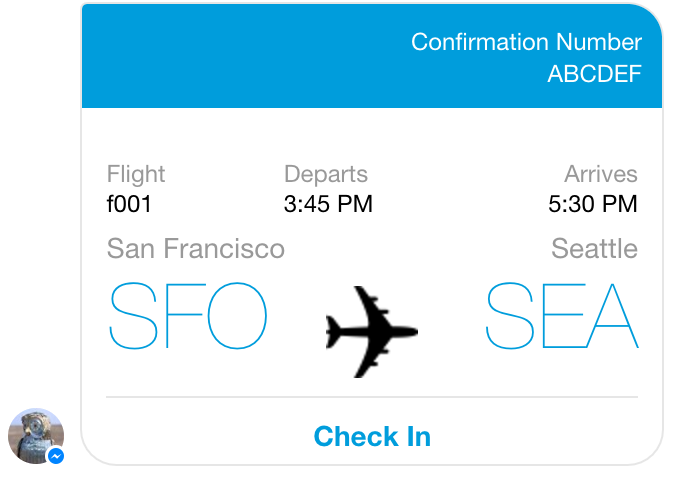
component: meya.airline_checkin
properties:
intro_message: Check-in is available now.
locale: en_US
pnr_number: ABCDEF
flight_info:
- flight_number: f001
departure_airport:
airport_code: SFO
city: San Francisco
terminal: T4
gate: G8
arrival_airport:
airport_code: SEA
city: Seattle
terminal: T4
gate: G8
flight_schedule:
boarding_time: 2016-01-05T15:05
departure_time: 2016-01-05T15:45
arrival_time: 2016-01-05T17:30
checkin_url: https://www.airline.com/check-in
from meyacards import AirlineCheckin
CHECKIN = {
"intro_message": "Check-in is available now.",
"locale": "en_US",
"pnr_number": "ABCDEF",
"flight_info": [
{
"flight_number": "f001",
"departure_airport": {
"airport_code": "SFO",
"city": "San Francisco",
"terminal": "T4",
"gate": "G8"
},
"arrival_airport": {
"airport_code": "SEA",
"city": "Seattle",
"terminal": "T4",
"gate": "G8"
},
"flight_schedule": {
"boarding_time": "2016-01-05T15:05",
"departure_time": "2016-01-05T15:45",
"arrival_time": "2016-01-05T17:30"
}
}
],
"checkin_url": "https://www.airline.com/check-in"
}
checkin_card = AirlineCheckin(payload=CHECKIN)
Airline Itinerary Template
Send a confirmation message that contains the itinerary and receipt.
See Facebook's documentation here
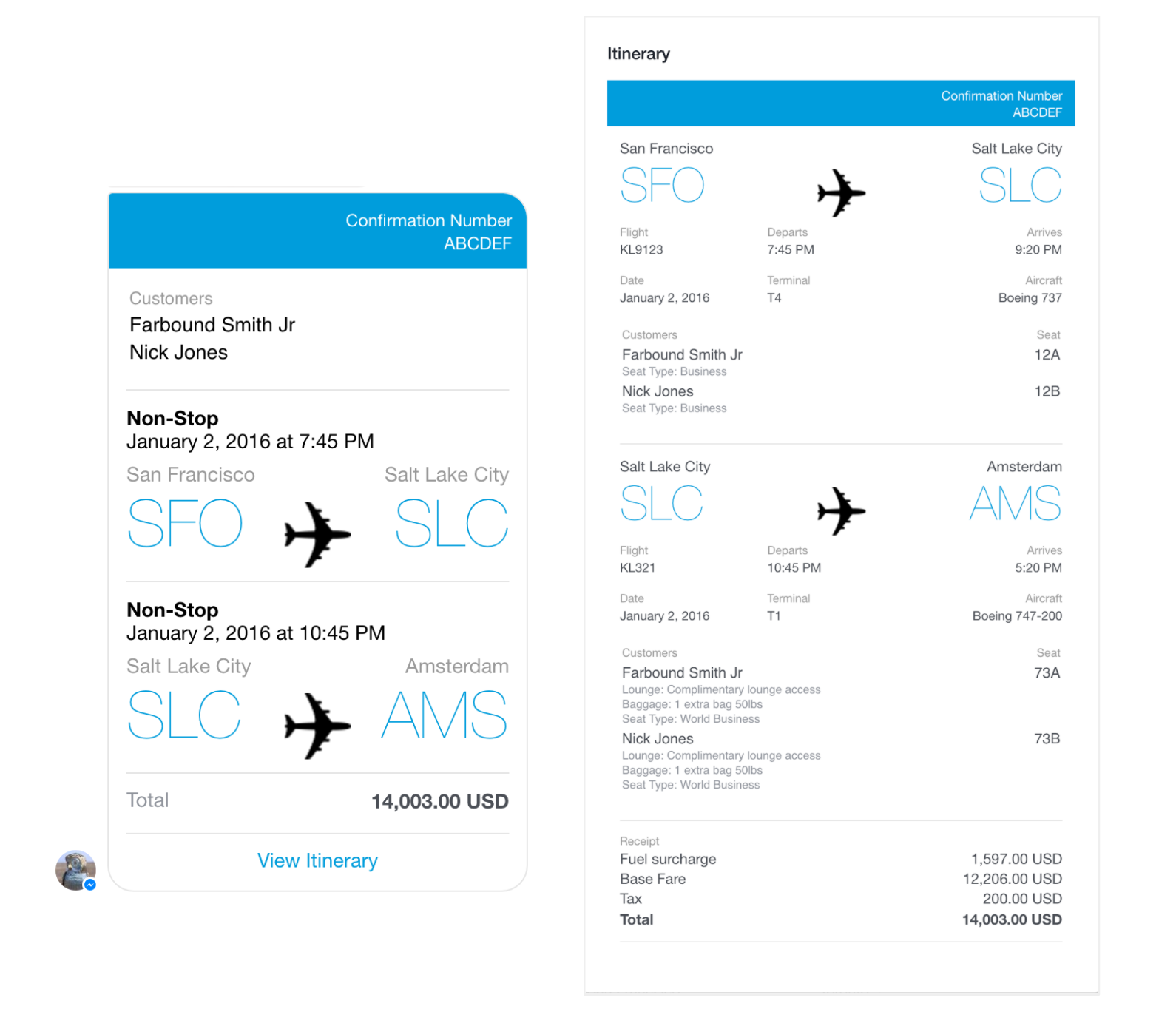
Itinerary message on left. Itinerary pop-up on right (after clicking "View Itinerary").
component: meya.airline_itinerary
properties:
intro_message: Here is your flight itinerary.
locale: en_US
pnr_number: ABCDEF
passenger_info:
- name: Farbound Smith Jr
ticket_number: 0741234567890
passenger_id: p001
- name: Nick Jones
ticket_number: 0741234567891
passenger_id: p002
flight_info:
- connection_id: c001
segment_id: s001
flight_number: KL9123
aircraft_type: Boeing 737
departure_airport:
airport_code: SFO
city: San Francisco
terminal: T4
gate: G8
arrival_airport:
airport_code: SLC
city: Salt Lake City
terminal: T4
gate: G8
flight_schedule:
departure_time: 2016-01-02T19:45
arrival_time: 2016-01-02T21:20
travel_class: business
- connection_id: c002
segment_id: s002
flight_number: KL321
aircraft_type: Boeing 747-200
travel_class: business
departure_airport:
airport_code: SLC
city: Salt Lake City
terminal: T1
gate: G33
arrival_airport:
airport_code: AMS
city: Amsterdam
terminal: T1
gate: G33
flight_schedule:
departure_time: 2016-01-02T22:45
arrival_time: 2016-01-03T17:20
passenger_segment_info:
- segment_id: s001
passenger_id: p001
seat: 12A
seat_type: Business
- segment_id: s001
passenger_id: p002
seat: 12B
seat_type: Business
- segment_id: s002
passenger_id: p001
seat: 73A
seat_type: World Business
product_info:
- title: Lounge
value: Complimentary lounge access
- title: Baggage
value: 1 extra bag 50lbs
- segment_id: s002
passenger_id: p002
seat: 73B
seat_type: World Business
product_info:
- title: Lounge
value: Complimentary lounge access
- title: Baggage
value: 1 extra bag 50lbs
price_info:
- title: Fuel surcharge
amount: '1597'
currency: USD
base_price: '12206'
tax: '200'
total_price: '14003'
currency: USD
from meyacards import AirlineItinerary
ITINERARY = {
"intro_message": "Here\'s your flight itinerary.",
"locale": "en_US",
"pnr_number": "ABCDEF",
"passenger_info": [
{
"name": "Farbound Smith Jr",
"ticket_number": "0741234567890",
"passenger_id": "p001"
},
{
"name": "Nick Jones",
"ticket_number": "0741234567891",
"passenger_id": "p002"
}
],
"flight_info": [
{
"connection_id": "c001",
"segment_id": "s001",
"flight_number": "KL9123",
"aircraft_type": "Boeing 737",
"departure_airport": {
"airport_code": "SFO",
"city": "San Francisco",
"terminal": "T4",
"gate": "G8"
},
"arrival_airport": {
"airport_code": "SLC",
"city": "Salt Lake City",
"terminal": "T4",
"gate": "G8"
},
"flight_schedule": {
"departure_time": "2016-01-02T19:45",
"arrival_time": "2016-01-02T21:20"
},
"travel_class": "business"
},
{
"connection_id": "c002",
"segment_id": "s002",
"flight_number": "KL321",
"aircraft_type": "Boeing 747-200",
"travel_class": "business",
"departure_airport": {
"airport_code": "SLC",
"city": "Salt Lake City",
"terminal": "T1",
"gate": "G33"
},
"arrival_airport": {
"airport_code": "AMS",
"city": "Amsterdam",
"terminal": "T1",
"gate": "G33"
},
"flight_schedule": {
"departure_time": "2016-01-02T22:45",
"arrival_time": "2016-01-03T17:20"
}
}
],
"passenger_segment_info": [
{
"segment_id": "s001",
"passenger_id": "p001",
"seat": "12A",
"seat_type": "Business"
},
{
"segment_id": "s001",
"passenger_id": "p002",
"seat": "12B",
"seat_type": "Business"
},
{
"segment_id": "s002",
"passenger_id": "p001",
"seat": "73A",
"seat_type": "World Business",
"product_info": [
{
"title": "Lounge",
"value": "Complimentary lounge access"
},
{
"title": "Baggage",
"value": "1 extra bag 50lbs"
}
]
},
{
"segment_id": "s002",
"passenger_id": "p002",
"seat": "73B",
"seat_type": "World Business",
"product_info": [
{
"title": "Lounge",
"value": "Complimentary lounge access"
},
{
"title": "Baggage",
"value": "1 extra bag 50lbs"
}
]
}
],
"price_info": [
{
"title": "Fuel surcharge",
"amount": "1597",
"currency": "USD"
}
],
"base_price": "12206",
"tax": "200",
"total_price": "14003",
"currency": "USD"
}
itinerary_card = AirlineItinerary(payload=ITINERARY)
Airline Flight Update Template
Send flight status update message..
See Facebook's documentation here
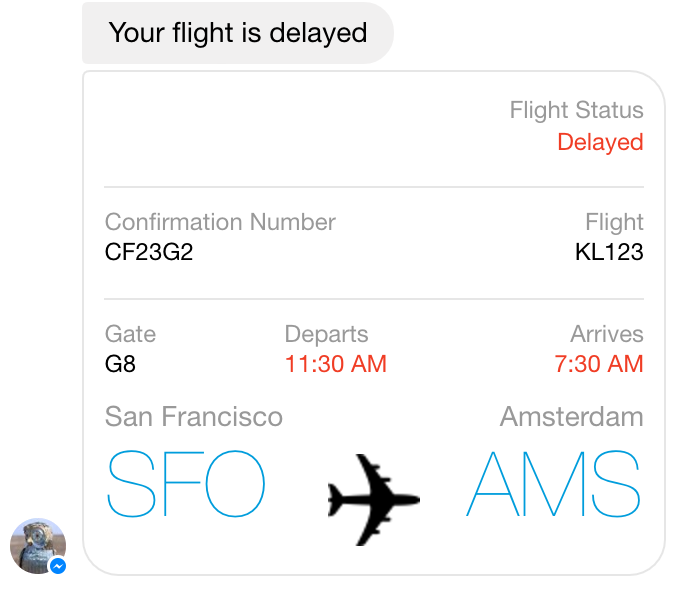
component: meya.airline_update
properties:
intro_message: Your flight is delayed
update_type: delay
locale: en_US
pnr_number: CF23G2
update_flight_info:
flight_number: KL123
departure_airport:
airport_code: SFO
city: San Francisco
terminal: T4
gate: G8
arrival_airport:
airport_code: AMS
city: Amsterdam
terminal: T4
gate: G8
flight_schedule:
boarding_time: 2015-12-26T10:30
departure_time: 2015-12-26T11:30
arrival_time: 2015-12-27T07:30
from meyacards import AirlineUpdate
UPDATE = {
"intro_message": "Your flight is delayed",
"update_type": "delay",
"locale": "en_US",
"pnr_number": "CF23G2",
"update_flight_info": {
"flight_number": "KL123",
"departure_airport": {
"airport_code": "SFO",
"city": "San Francisco",
"terminal": "T4",
"gate": "G8"
},
"arrival_airport": {
"airport_code": "AMS",
"city": "Amsterdam",
"terminal": "T4",
"gate": "G8"
},
"flight_schedule": {
"boarding_time": "2015-12-26T10:30",
"departure_time": "2015-12-26T11:30",
"arrival_time": "2015-12-27T07:30"
}
}
}
update_card = AirlineUpdate(payload=UPDATE)
Updated about 2 months ago